JavaScript Object Notation
|
Read other articles:

Tugu Kemenangan Berlin di Tiergarten. Tugu Kemenangan Berlin (Bahasa Jerman: Berliner Siegessäule) adalah monumen yang terletak di jantung kota Berlin, Jerman. Tugu ini tepatnya berada di taman kota Tiergarten dan dekat dari ikon kota Berlin yaitu Gerbang Bradenburg. Desain monumen ini dibuat oleh Heinrich Strack, konstruksi pertama dibangun pada tahun 1864 untuk memperingati kemenangan Prusia atas Denmark dalam Perang Prusia-Denmark (1 Februari – 30 Oktober 1864).[1][2] da...

Dalam cerita Alkitab Tangga Yakub, Yakub bermimpi soal tangga menuju surga. Seorang karakter yang memiliki mimpi adalah cara umum untuk menambahkan cerita dalam pada cerita yang lebih besar. (Lukisan karya William Blake, 1805) Cerita dalam cerita, juga disebut sebagai cerita selipan, adalah sebuah perangkat sastra dimana sebuah karakter dalam sebuah cerita menjadi narator cerita kedua (dalam cerita pertama).[1] Lapisan cerita berganda dalam cerita terkadang disebut cerita bersarang. S...

United States historic placeH. E. Gensky Grocery Store BuildingU.S. National Register of Historic Places Show map of MissouriShow map of the United StatesLocation423 Cherry St., Jefferson City, MissouriCoordinates38°34′7″N 92°9′54″W / 38.56861°N 92.16500°W / 38.56861; -92.16500Arealess than one acreBuilt1915 (1915)Built bySchmidli, JosephArchitectural styleEarly CommercialNRHP reference No.01000628[1]Added to NRHPJune 6, 2001 H. ...

Paruh-perak Euodice Indian silverbill (Euodice malabarica)TaksonomiKerajaanAnimaliaFilumChordataKelasAvesOrdoPasseriformesFamiliEstrildidaeGenusEuodice Reichenbach, 1862 SpeciesSee text.lbs Euodice atau paruh-perak adalah genus burung pemakan biji kecil dalam keluarga Estrildidae . Spesies ini berasal dari zona kering di Afrika dan India dan biasa. Mereka dahulunya termasuk dalam genus Lonchura . Taksonomi Image Common Name Scientific name Distribution Paruh-perak Afrika Euodice cantans Centr...

Peta perubahan wilayah Amerika Serikat. Amerika Serikat terletak di tengah-tengah benua Amerika Utara, dibatasi oleh Kanada di sebelah utara dan Meksiko di sebelah selatan. Negara Amerika Serikat terbentang dari Samudra Atlantik di pesisir timur hingga Samudra Pasifik di pesisir barat, termasuk kepulauan Hawaii di lautan Pasifik, negara bagian Alaska di ujung utara benua Amerika, dan beberapa teritori lainnya. Penetap pertama wilayah yang kini menjadi Amerika Serikat berasal dari Asia sekitar...
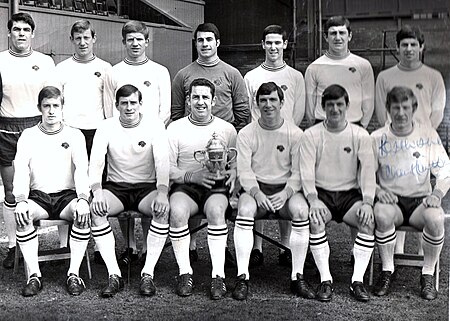
Scottish footballer For the actor, see John O'Hare (actor). John O'HarePersonal informationDate of birth (1946-09-24) 24 September 1946 (age 77)Place of birth Renton, ScotlandPosition(s) ForwardYouth career1963–1964 SunderlandSenior career*Years Team Apps (Gls)1964–1967 Sunderland 51 (14)1967 Vancouver Royal Canadians 11 (1)1967–1974 Derby County 248 (65)1974–1975 Leeds United 6 (1)1975–1981 Nottingham Forest 101 (14)1977–1978 Dallas Tornado 40 (14) Belper Town ? (?)Total 41...

Circuit de Reims-GueuxLokasiGueux, FranceZona waktuGMT +1Acara besarGrand Prix de la MarneFrench Grand Prix12 Hours of Reims,1926 Original circuitPanjang7.816 km (4.856 mi)Tikungan8Rekor lap2:27.8 ( Juan Manuel Fangio, Alfa Romeo 159, 1951, Formula One)1952 VariationPanjang7.152 km (4.444 mi)Tikungan51953 VariationPanjang8.372 km (5.187 mi)Tikungan7Rekor lap2:41.0 ( Juan Manuel Fangio, Maserati, 1953, Formula One)1954 VariationPanjang8.302 km (5.158 mi)Tikungan7Rekor lap2:11.3 ...

2004 Air Canada CupTournament detailsHost country GermanyDates5 – 7 February 2004Teams4Final positionsChampions Canada U22 (2nd title)Runner-up GermanyThird place SwitzerlandTournament statisticsGames played6← 20032005 → The 2004 Air Canada Cup was the second edition of the women's ice hockey tournament. It was held from February 5-7, 2004 in Garmisch-Partenkirchen and Bad Tölz, Germany. The Canadian U22 national team w...
Le informazioni riportate non sono consigli medici e potrebbero non essere accurate. I contenuti hanno solo fine illustrativo e non sostituiscono il parere medico: leggi le avvertenze. Muscolo gemello inferioreSi mostrano i Gruppo dei rotatori laterali dell'anca, fra cui il muscolo gemello inferioreAnatomia del Gray(EN) Pagina 477 Originetuberosità ischiatica Inserzionetrochanteric fossa Azioniextrarotazione dell'anca Arteriaarteria gluteale inferiore Nervonervo dei muscoli quadrato del fem...

2009 single by Jay Sean Do You RememberSingle by Jay Sean featuring Sean Paul and Lil Jonfrom the album All or Nothing and the mixtape The Odyssey Mixtape Released3 November 2009RecordedDecember 2008Length3:30Label Jayded 2Point9 Cash Money Universal Republic Songwriter(s) Kamaljit Singh Jhooti Sean Paul Henriques Jonathan Smith Jared Cotter Frankie Storm J-Remy Bobby Bass Jonathan Perkins Producer(s)J-Remy & Bobby BassJay Sean singles chronology Written on Her (2009) Do You Remember ...

1975 South Korean film directed by Kim Ho-sun Yeong-ja's HeydaysDirected byKim Ho-sunWritten byJo Seon-jakProduced byKim Tai-sooStarringYeom Bok-sun Song Jae-hoCinematographyJang Seok-junEdited byYoo Jae-wonMusic byJeong Sung-joRelease date February 11, 1975 (1975-02-11) Running time103 minutesCountrySouth KoreaLanguageKorean Yeong-ja's Heydays (Korean: 영자의 전성시대; RR: Yeongja-ui jeonseong sidae) is a 1975 South Korean film directed by Kim Ho-s...
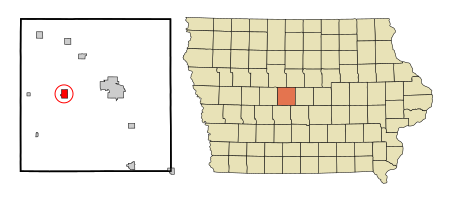
لمعانٍ أخرى، طالع أوغدن (توضيح). أوغدن الإحداثيات 42°02′24″N 94°01′50″W / 42.04°N 94.030555555556°W / 42.04; -94.030555555556 [1] تقسيم إداري البلد الولايات المتحدة[2] التقسيم الأعلى مقاطعة بون خصائص جغرافية المساحة 3.538326 كيلومتر مربع3.557336 كيلومتر مر�...

Canadian politician (born 1964) The HonourableCandice BergenPCBergen in 2017Leader of the OppositionIn officeFebruary 2, 2022 – September 10, 2022MonarchsElizabeth IICharles IIIDeputyLuc BertholdPreceded byErin O'TooleSucceeded byPierre PoilievreInterim Leader of the Conservative PartyIn officeFebruary 2, 2022 – September 10, 2022PresidentRobert BathersonDeputyLuc BertholdPreceded byErin O'TooleSucceeded byPierre PoilievreDeputy Leader of the OppositionIn officeSeptember...
Il figlio di DraculaTitolo originaleSon of Dracula Lingua originaleinglese Paese di produzioneStati Uniti d'America Anno1943 Durata80 min Dati tecnicib/n Genereorrore RegiaRobert Siodmak Ford Beebe (regista 2ª unità) SceneggiaturaEric Taylor ProduttoreFord Beebe Casa di produzioneUniversal Pictures TruccoJack Pierce Interpreti e personaggi Lon Chaney Jr.: Il conte Alucard J. Edward Bromberg: professor Lazlo Robert Paige: Frank Stanley Louise Allbritton: Katherine Caldwell Evelyn Ankers: Cla...
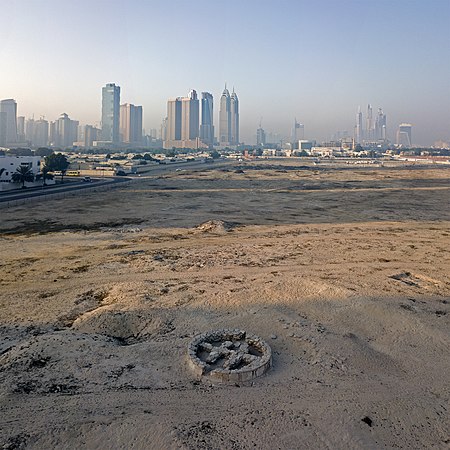
This article is part of a series on the History of the United Arab Emirates Bronze Age Magan civilization Umm Al Nar culture Archaeological sites Mleiha Al Ashoosh Al Sufouh Ed-Dur Hili Saruq Al Hadid Shimal Tell Abraq Iron Age Wadi Suq culture Archaeological sites Al Thuqeibah Bidaa Bint Saud Ed-Dur Muweilah Seih Al Harf Qattara Oasis Rumailah Saruq Al Hadid Shimal Tell Abraq Pre-Islamic Era Sasanian rule Archaeological sites Ed-Dur Islamic Era Battle of Dibba Colonial Era Portuguese Dibba ...

Room where administrative work is performed For other uses, see Office (disambiguation). This article needs additional citations for verification. Please help improve this article by adding citations to reliable sources. Unsourced material may be challenged and removed.Find sources: Office – news · newspapers · books · scholar · JSTOR (May 2024) (Learn how and when to remove this message) Midtown Manhattan in New York City is the largest central busine...

The examples and perspective in this article may not represent a worldwide view of the subject. You may improve this article, discuss the issue on the talk page, or create a new article, as appropriate. (August 2021) (Learn how and when to remove this message) Chlorpyrifos Names Preferred IUPAC name O,O-Diethyl O-(3,5,6-trichloropyridin-2-yl) phosphorothioate Other names Brodan, Bolton insecticide, Chlorpyrifos-ethyl, Cobalt, Detmol UA, Dowco 179, Dursban, Empire, Eradex, Hatchet, Lorsban, Nu...
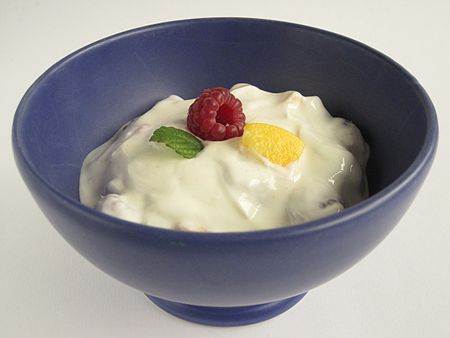
Indonesian traditional fermented milk DadiahPlace of originIndonesiaRegion or stateWest SumatraMain ingredientsBuffalo milk Dadiah (Minangkabau) or dadih (Indonesian and Malaysian Malay) a traditional fermented milk popular among people of West Sumatra, Indonesia, is made by pouring fresh, raw, unheated, buffalo milk into a bamboo tube capped with a banana leaf and allowing it to ferment spontaneously at room temperature for two days. The milk is fermented by indigenous lactic bacteria found ...

Gendarmerie of the Democratic Republic of Afghanistan SarandoySarandoy gendarmes at a checkpoint (LIFE magazine)Active1978–1992CountryAfghanistanAllegianceDemocratic Republic of AfghanistanBranchMinistry of Interior AffairsTypeGendarmerieRoleReserve armyInternal securityAnti-tank warfareCounter-insurgencyCounter-intelligenceCovert operationDesert warfareMountain warfareExecutive protectionForce protectionHUMINTLaw enforcementManhuntRaidingReconnaissanceSecurity checkpointTrackingTraffic pol...
Art school at the University of Oregon This article may rely excessively on sources too closely associated with the subject, potentially preventing the article from being verifiable and neutral. Please help improve it by replacing them with more appropriate citations to reliable, independent, third-party sources. (December 2014) (Learn how and when to remove this message) University of Oregon College of DesignLawrence Hall, the main building of UO DesignTypePublicEstablished1914DeanAdrian Par...