Kotlin
|
Read other articles:

Об экономическом термине см. Первородный грех (экономика). ХристианствоБиблия Ветхий Завет Новый Завет Евангелие Десять заповедей Нагорная проповедь Апокрифы Бог, Троица Бог Отец Иисус Христос Святой Дух История христианства Апостолы Хронология христианства Ран�...

Constituency of Bangladesh's Jatiya Sangsad Tangail-7Constituencyfor the Jatiya SangsadDistrictTangail DistrictDivisionDhaka DivisionElectorate322,673 (2018)[1]Current constituencyCreated1973PartyAwami LeagueMember(s)Khan Ahmed Shuvo Tangail-7 is a constituency represented in the Jatiya Sangsad (National Parliament) of Bangladesh by Khan Ahmed Shuvo. Since 2001 it was represented by Md. Akabbar Hossain of the Awami League. Boundaries The constituency encompasses Mirzapur Upazila.[...

Синелобый амазон Научная классификация Домен:ЭукариотыЦарство:ЖивотныеПодцарство:ЭуметазоиБез ранга:Двусторонне-симметричныеБез ранга:ВторичноротыеТип:ХордовыеПодтип:ПозвоночныеИнфратип:ЧелюстноротыеНадкласс:ЧетвероногиеКлада:АмниотыКлада:ЗавропсидыКласс:Пт�...

Pour la commune, voir Thérouanne. la Thérouanne La Thérouanne à Douy-la-Ramée. la Thérouanne sur OpenStreetMap. Caractéristiques Longueur 23,3 km [1] Bassin 167 km2 [2] Bassin collecteur la Seine Débit moyen 0,601 m3/s (Congis-sur-Thérouanne(Gué-à-Tresmes)) [2] Organisme gestionnaire SMAERTA ou Syndicat Mixte d’Aménagement et d’Entretien de la Rivière Thérouanne et de ses Affluents[3] Régime pluvial océanique Cours Source source · Localisation Saint-Pathus...

Group of growth factor proteins Bone morphogenetic proteins (BMPs) are a group of growth factors also known as cytokines and as metabologens.[1] Originally discovered by their ability to induce the formation of bone and cartilage, BMPs are now considered to constitute a group of pivotal morphogenetic signals, orchestrating tissue architecture throughout the body.[2] The important functioning of BMP signals in physiology is emphasized by the multitude of roles for dysregulated ...
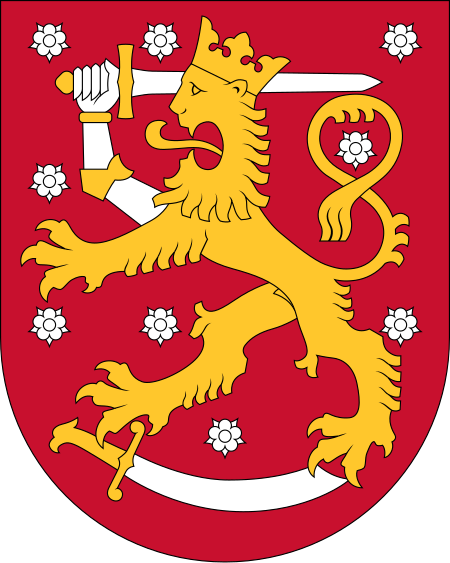
Administrative subdivisions formed in 2009 You can help expand this article with text translated from the corresponding article in Finnish. (June 2023) Click [show] for important translation instructions. Machine translation, like DeepL or Google Translate, is a useful starting point for translations, but translators must revise errors as necessary and confirm that the translation is accurate, rather than simply copy-pasting machine-translated text into the English Wikipedia. Do not tran...
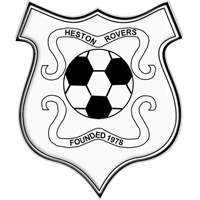
Football clubHeston RoversNickname(s)The Zodiac LunchesFounded1978GroundPalmerston Park, DumfriesCapacity8,690 (3,377 seated)ChairmanAlan A WatsonManagerMichael Houston2020–21South of Scotland League (season abandoned) Home colours Away colours Heston Rovers Football Club are a Scottish senior football club who play their home games at Palmerston Park in Dumfries, which they share with Queen of the South. Heston is a member of the South of Scotland Football League and D&G Youth Footbal...

GPIB Jemaat Sion DKI JakartaGereja Protestan di Indonesia bagian BaratGedung Gereja Sion, JakartaLokasiJakarta, IndonesiaDenominasiProtestanArsitekturStatus fungsionalAktifPenetapan warisanATipe arsitekturGereja Cagar budaya IndonesiaGereja Sion JakartaPeringkatNasionalKategoriBangunanNo. RegnasCB.625LokasikeberadaanJakarta Barat, DKI JakartaNo. SK193/M/2017Tanggal SK14 Juli 2017Tingkat SKMenteriPemilikGereja Protestan Indonesia Bagian BaratPengelolaGereja Protestan Indonesia Bagian Barat Jem...

豪栄道 豪太郎 場所入りする豪栄道基礎情報四股名 澤井 豪太郎→豪栄道 豪太郎本名 澤井 豪太郎愛称 ゴウタロウ、豪ちゃん、GAD[1][2]生年月日 (1986-04-06) 1986年4月6日(38歳)出身 大阪府寝屋川市身長 183cm体重 160kgBMI 47.26所属部屋 境川部屋得意技 右四つ・出し投げ・切り返し・外掛け・首投げ・右下手投げ成績現在の番付 引退最高位 東大関生涯戦歴 696勝493敗...

Cet article est une ébauche concernant une localité iranienne. Vous pouvez partager vos connaissances en l’améliorant (comment ?) selon les recommandations des projets correspondants. Bandar Abbas (fa) بندر عباس Administration Pays Iran Province Hormozgan Indicatif téléphonique international +(98) Démographie Population 526 648 hab. (2016) Géographie Coordonnées 27° 11′ 11″ nord, 56° 16′ 38″ est Altitude 7 m Locali...

Albania Estación miembro Final Nacional Festivali I Këngës Apariciones 21 (2024) Primera aparición 2004 Mejor resultado Final 5.º 2012 Semifinal 2.º 2012 Peor resultado Final 22.º 2023 Semifinal 17.º 2007 Enlaces externos Sitio oficial de RTSH Página de Albania en Eurovision.tv Albania ha participado en el Festival de la Canción de Eurovisión un total de diecisiete ocasiones, desde su debut en la edición de 2004, en la que Anjeza Shahini terminó en séptimo lugar con la canción...

County in Mississippi, United States County in MississippiWarren CountyCountyWarren County Courthouse in Vicksburg, built c. 1940, located across from the Old Courthouse Museum.Location within the U.S. state of MississippiMississippi's location within the U.S.Coordinates: 32°22′N 90°51′W / 32.36°N 90.85°W / 32.36; -90.85Country United StatesState MississippiFoundedDecember 22, 1809Named forJoseph WarrenSeatVicksburgLargest cityVicksburgArea •...

Professional sports hall of fame in New York, U.S.Sports Museum of AmericaEstablishedMay 7, 2008; 16 years ago (2008-05-07)DissolvedFebruary 20, 2009 (2009-02-20)Location26 Broadway (Standard Oil Building), Manhattan, New York City, New York, U.S.TypeProfessional sports hall of fameAccreditationFor-profitCollection size1,100 photographs and 800 artifactsVisitors125,000FounderPhilip Schwalb and Sameer AhujaCEOPhilop SchwalbOwnerMeaningful Entertainment Group ...

Musical instrument Andrea Neumann's preparations, where pieces of cutlery are placed between piano strings Phillip Zoubek's prepared piano A prepared piano is a piano that has had its sounds temporarily altered by placing bolts, screws, mutes, rubber erasers, and/or other objects on or between the strings. Its invention is usually traced to John Cage's dance music for Bacchanale (1940), created for a performance in a Seattle venue that lacked sufficient space for a percussion ensemble. Cage h...

British peer This article needs additional citations for verification. Please help improve this article by adding citations to reliable sources. Unsourced material may be challenged and removed.Find sources: Richard Rhys, 9th Baron Dynevor – news · newspapers · books · scholar · JSTOR (January 2021) (Learn how and when to remove this message) Richard Charles Uryan Rhys, 9th Baron Dynevor (19 June 1935 – 12 November 2008) was a British peer. He was ed...

Sanal EdamarukuDikenal atasPresiden Indian Rationalist AssociationSanal Edamaruku adalah pendiri dan presiden Rationalist International.[1] Ia juga merupakan presiden Indian Rationalist Association. Ia adalah penyunting publikasi internet Rationalist International, serta pengarang 25 buku dan banyak artikel. Pada tahun 2012, dituduh melukai perasaan religius karena membantah klaim mukjizat di Gereja Katolik lokal. Kehidupan Kehidupan awal Ia lahir pada tahun 1955 di Thodupuzha, Keral...
هذه المقالة يتيمة إذ تصل إليها مقالات أخرى قليلة جدًا. فضلًا، ساعد بإضافة وصلة إليها في مقالات متعلقة بها. (يونيو 2019) أجيم رمضاني معلومات شخصية الميلاد 3 مايو 1964 الوفاة 11 أبريل 1999 (34 سنة) مواطنة ألبانيا الحياة العملية المهنة كاتب اللغات الألبانية الخدمة �...

Ne doit pas être confondu avec La Chaîne parlementaire. Pour les articles homonymes, voir LCP. LCP - Assemblée nationaleCaractéristiquesCréation 21 mars 2000Propriétaire Assemblée nationaleSlogan « Donnons du sens »Format d'image 576i (SDTV) et 1080i (HDTV)Langue FrançaisPays FranceStatut Chaîne publique d'information parlementaire et politiqueSiège social 106, rue de l'Université75007 ParisSite web lcp.frDiffusionNumérique TNT : chaîne no 13 (temps partiel...
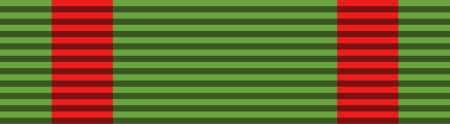
Italian footballer and manager Walter Zenga Zenga coaching Wolverhampton Wanderers in 2016Personal informationFull name Walter Zenga[1]Date of birth (1960-04-28) 28 April 1960 (age 64)Place of birth Milan, ItalyHeight 1.88 m (6 ft 2 in)Position(s) GoalkeeperYouth career1969–1971 Macallesi 19271971–1978 Inter MilanSenior career*Years Team Apps (Gls)1978–1994 Inter Milan 328 (0)1978–1979 → Salernitana (loan) 3 (0)1979–1980 → Savona (loan) 23 (0)1980–1...

Occupation of derelict land or abandoned buildings Philippines on globe A squatter settlement in Manila in 2007 Shacks at Payatas dumpsite in 2010 Urban areas in the Philippines such as Metro Manila, Metro Cebu, and Metro Davao have large informal settlements. The Philippine Statistics Authority defines a squatter, or alternatively informal dwellers, as One who settles on the land of another without title or right or without the owner's consent whether in urban or rural areas.[1] Squa...