スレッド局所記憶
|
Read other articles:

Часть серии статей о Холокосте Идеология и политика Расовая гигиена · Расовый антисемитизм · Нацистская расовая политика · Нюрнбергские расовые законы Шоа Лагеря смерти Белжец · Дахау · Майданек · Малый Тростенец · Маутхаузен ·&...

Torsten Frings Frings bersama Werder tahun 2007.Informasi pribadiNama lengkap Torsten FringsTanggal lahir 22 November 1976 (umur 47)Tempat lahir Würselen, Jerman BaratTinggi 1,82 m (5 ft 11+1⁄2 in)Posisi bermain Gelandang bertahanInformasi klubKlub saat ini Toronto FCNomor 22Karier junior1982–1988 Rot-Weiß Alsdorf1988–1990 Rhenania Alsdorf1990–1994 Alemannia AachenKarier senior*Tahun Tim Tampil (Gol)1994–1997 Alemannia Aachen 56 (13)1997 Werder Bremen (A) ...

La radio AM est un procédé de radiodiffusion à partir d'émetteurs utilisant la modulation d'amplitude. Ce terme désigne également le récepteur qui permet son écoute. La radio AM est utilisée dans les bandes de radiodiffusion GO, PO et OC, où elle permet une couverture plus large que la radio FM, avec une largeur de bande réduite. Modulation d'amplitude Spectre en fréquence d'une modulation d'amplitude Largeur de bande La modulation d'amplitude est la variation en amplitude d'un si...

Staphylococcus aureus - Plat Uji Antibiotik Antibiotik adalah golongan senyawa antimikroba yang mempunyai efek menekan atau menghentikan suatu proses biokimia pada organisme, khususnya dalam proses infeksi oleh bakteri.[1] Penggunaan antibiotik khususnya berkaitan dengan pencegahan dan pengobatan penyakit infeksi, termasuk bahan antibakteri paling penting.[2][3] Antibiotik bekerja dengan mematikan atau menghalangi pertumbuhan populasi bakteri. Sejumlah antibiotik juga ...

Since 2013, radio coverage of the Cleveland Browns professional football team has originated from flagship stations WKNR (850 AM), WKRK-FM (92.3 FM) and WNCX (98.5 FM). Jim Donovan has served as the team's play-by-play announcer since it resumed play in 1999. Color commentator Nathan Zegura and sideline analyst/reporter Je'Rod Cherry round out the radio team.[1] Spanish language broadcasts are heard on WNZN 89.1 FM with announcers Rafa Hernández-Brito and Octavio Sequera. WEWS (chan...

Elena Zareschi nel 1965 Elena Zareschi, pseudonimo di Elina Lazzareschi (Buenos Aires, 23 giugno 1916 – Lucca, 31 luglio 1999), è stata un'attrice italiana. Indice 1 Biografia 2 Prosa teatrale 3 Filmografia 3.1 Doppiatrici 4 Doppiaggio 5 Prosa radiofonica Rai 6 Prosa televisiva Rai 7 Altri progetti 8 Collegamenti esterni Biografia Nata in Argentina da una famiglia di commercianti toscani di origine lucchese, arriva in Italia alla metà degli anni trenta, si presenta alle selezioni per freq...

Cet article est une ébauche concernant la radiodiffusion et les États-Unis. Vous pouvez partager vos connaissances en l’améliorant (comment ?) selon les pratiques du projet Radio. BBC Asian Network Présentation Pays Royaume-Uni Siège social Birmingham (The Mailbox) et Londres (Broadcasting House) Propriétaire British Broadcasting Corporation Langue Anglais+ 5 langues asiatiques Différents noms The Asian Network (1988-1996) Historique Création 30 octobre 1988 (temps partagé av...

Peta pulau-pulau di Tonga Pembagian administratif Tonga terdiri atas 5 divisi pada tingkat pertama dan 23 distrik pada tingkat kedua. lbsPembagian administratif OseaniaNegara berdaulat Australia Federasi Mikronesia Fiji Kepulauan Marshall Kepulauan Solomon Kiribati Nauru Palau Papua Nugini Samoa Selandia Baru Timor Leste1 Tonga Tuvalu Vanuatu Dependensi danwilayah lain Kepulauan Cocos (Keeling) Kepulauan Cook Guam Hawaii Kaledonia Baru Kepulauan Mariana Utara Pulau Natal Niue Pulau Norfolk Pu...

Galileo di hadapan sidang Inkuisisi Roma Inkuisisi (dengan huruf I besar) adalah istilah yang secara luas digunakan untuk menyebut pengadilan terhadap ahli bidat oleh Gereja Katolik Roma. Istilah ini juga dapat bermakna tribunal gerejawi atau lembaga dalam Gereja Katolik Roma yang bertugas melawan atau menyingkirkan bidat, sejumlah gerakan ekspurgasi historis terhadap bidat (yang digiatkan oleh Gereja Katolik Roma), atau pengadilan atas seseorang yang didakwa bidat[1] Definisi dan tuj...

Nazaruddin Sjamsuddin [[Ketua Komisi Pemilihan Umum Republik Indonesia]] 2Masa jabatan2001–2005PresidenAbdurrahman WahidPendahuluRudiniPenggantiAbdul Hafiz Anshari(pada tahun 2007) Informasi pribadiLahir5 November 1944 (umur 79)Kota Juang, Bireuen, Aceh, IndonesiaSunting kotak info • L • B Prof. Dr. Nazaruddin Sjamsuddin, MA (lahir 5 November 1944) adalah mantan Ketua Komisi Pemilihan Umum (KPU) yang bertugas memantau jalannya Pemilu di Indonesia untuk periode 2001-20...

New Zealand freestyle skier Margaux HackettPersonal informationBorn (1999-06-02) 2 June 1999 (age 24)Annecy, FranceRelativeA. J. Hackett (father)SportCountryNew ZealandSportFreestyle skiing Margaux Hackett (born 2 June 1999) is a New Zealand freestyle skier who competes internationally.[1] She represented New Zealand in the slopestyle and big air events at the 2022 Winter Olympics in Beijing, China.[2] Biography Hackett was born and raised in Annecy, France, to a New Zeal...

Questa voce o sezione sull'argomento esploratori spagnoli non cita le fonti necessarie o quelle presenti sono insufficienti. Puoi migliorare questa voce aggiungendo citazioni da fonti attendibili secondo le linee guida sull'uso delle fonti. Spedizione di Juan de Oñate Tipoterrestre Parte diColonizzazione spagnola delle Americhe Obiettivocolonizzare la parte settentrionale del Rio Grande Anni1595 EsitoFondata la provincia di Santa Fe de Nuevo México ConseguenzeNominato primo governator...

1926 agreement between the United States and France Mellon-Berenger AgreementSigned29 April 1926 (1926-04-29)LocationWashington, D.C., ParisEffective18 December 1929 (1929-12-18)Negotiators Andrew W. Mellon Henry Bérenger Parties United States France Languages French English The Mellon-Berenger Agreement (or Accord Mellon-Bérenger) (29 April 1926) was an agreement on the amount and rate of repayment of France's debt to the United States arising from loans and p...

Systemic herbicide and crop desiccant This article is about the chemical alone. For herbicides based on it, see Glyphosate-based herbicides. For the brand-name formulation developed by Monsanto, see Roundup (herbicide). Not to be confused with Glufosinate. Glyphosate Idealised skeletal formula Ball-and-stick model of the zwitterion based on the crystal structure[1][2] Names Pronunciation /ˈɡlɪfəseɪt, ˈɡlaɪfə-/,[3] /ɡlaɪˈfɒseɪt/[4][5] IUPAC...

Australian racing driver and politician (born 1942) Allan Grice OAMAllan-Chris Grice at Amaroo Park RacewayBorn (1942-10-21) 21 October 1942 (age 81)Maitland, New South WalesNationality AustralianOccupationracing driverRetired2005V8 UtesYears active2002–05TeamsAllan Grice RacingBest finish6th in 2002 V8 Brutes SeriesPrevious series1966, 197219721974–951978–801981–851981–9119841984, 19881986, 198819861987, 198919871988198819881989–19912002–2005Australian Drivers' Championshi...

This article has multiple issues. Please help improve it or discuss these issues on the talk page. (Learn how and when to remove these template messages) This article may be in need of reorganization to comply with Wikipedia's layout guidelines. Please help by editing the article to make improvements to the overall structure. (July 2015) (Learn how and when to remove this message) This article needs additional citations for verification. Please help improve this article by adding citations t...

Questa voce o sezione sull'argomento competizioni calcistiche non è ancora formattata secondo gli standard. Commento: Voce da adeguare al modello di voce per una stagione di campionato di calcio Contribuisci a migliorarla secondo le convenzioni di Wikipedia. Segui i suggerimenti del progetto di riferimento. 2. Fußball-Bundesliga 2016-2017 Competizione 2. Fußball-Bundesliga Sport Calcio Edizione 43ª Organizzatore DFB Date dal 5 agosto 2016al 21 maggio 2017 Luogo Germani...

System resource identifier in operating systems In Unix and Unix-like computer operating systems, a file descriptor (FD, less frequently fildes) is a process-unique identifier (handle) for a file or other input/output resource, such as a pipe or network socket. File descriptors typically have non-negative integer values, with negative values being reserved to indicate no value or error conditions. File descriptors are a part of the POSIX API. Each Unix process (except perhaps daemons) should ...

This article has multiple issues. Please help improve it or discuss these issues on the talk page. (Learn how and when to remove these messages) This article needs additional citations for verification. Please help improve this article by adding citations to reliable sources. Unsourced material may be challenged and removed.Find sources: Violino piccolo – news · newspapers · books · scholar · JSTOR (April 2021) (Learn how and when to remove this messag...
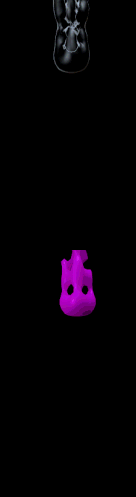
Confronto tra il comportamento di due sostanze aventi differente viscosità (in alto: sostanza a viscosità minore; in basso: sostanza a viscosità maggiore). Nell'ambito dei fenomeni di trasporto, la viscosità è il coefficiente di scambio di quantità di moto.[N 1] Dal punto di vista microscopico la viscosità è legata all'attrito tra le molecole del fluido. Quando il fluido è fatto scorrere dentro una tubatura, le particelle che compongono il fluido generalmente si muovono più ...