M-tree
|
Read other articles:

1848 Circulating coins Face value Coin Obverse design Reverse design Composition Mintage Available Obverse Reverse $2.50 CAL Liberty quarter eagle [Note 1] Standard Liberty Head quarter eagle obverse Standard Liberty Head quarter eagle reverse with CAL. punched into the field 90% Au, 10% Cu Uncirculated: 1,389 (P)[1] 1848 1892 Non-circulating coins Face value Coin Obverse design Reverse design Composition Mintage Available Obverse Reverse 50¢ Columbian half dollar Christopher Columb...

Serbian newspaper BlicTypeDaily newspaperFormatTabloidOwner(s)RingierPublisherRingier Serbia doo.EditorMarko StjepanovićFounded16 September 1996; 27 years ago (1996-09-16)Political alignmentPopulismCivic nationalismSensationalismAnti-Russian sentiment[1]HeadquartersŽorža Klemansoa 19, Belgrade, SerbiaCirculation~58,000 copies sold (2016)Websiteblic.rs Blic (Cyrillic: Блиц, [ˈbliːt͡s]) is a daily middle-market tabloid newspaper in Serbia. Founded in ...

Strada statale 449di Diano MarinaDenominazioni successiveStrada provinciale 449 LocalizzazioneStato Italia Regioni Liguria DatiClassificazioneStrada statale InizioDiano Marina FineImperia Lunghezza3,796[1] km Provvedimento di istituzioneD.M. 15/09/1965 - G.U. 274 del 2/11/1965[2] GestoreTratte ANAS: nessuna (dal 2001 la gestione è passata alla Provincia di Imperia) Manuale La ex strada statale 449 di Diano Marina (SS 449), ora strada provinciale 449, è una strada p...

Indian trade unionist and politician (1930–2019) For the American soccer player, see George Fernandez. George FernandesGeorge Fernandes in 200222nd Minister of DefenceIn office21 October 2001 – 22 May 2004Prime MinisterAtal Bihari VajpayeePreceded byJaswant SinghSucceeded byPranab MukherjeeIn office19 March 1998 – 16 March 2001Prime MinisterAtal Bihari VajpayeePreceded byMulayam Singh YadavSucceeded byJaswant SinghMinister of RailwaysIn office2 December 1989 –...

Pooja BosePooja Bose pada rilis audio Rajdhani ExpressLahir06 Februari 1987 (umur 37)Kolkata, India.KebangsaanIndianPekerjaanAktris, modelSuami/istriArnoy Chakraborty [1] Pooja Bose adalah seorang aktris televisi India.[2][3] Dia terkenal karena bermain Vrinda di pertunjukan populer Tujh Sang Preet Lagai Sajna, yang ditayangkan di Star Plus. Dia adalah seorang kontestan Jhalak Dikhhla Jaa pada tahun 2014 Dan Comedy Nights Bachao pada tahun 2015. Dia saat ini terl...

Untuk organisasi bernama Komite Bumi Putera atau Comite Boemi Poetera, lihat Boemi Poetera. Masyarakat bumiputera di Malaysia. Bumiputera atau Bumiputra (dari bahasa Sanskerta) adalah istilah resmi yang digunakan luas di Malaysia dan Brunei untuk merujuk kepada suku Melayu dan juga kelompok pribumi lainnya. Definisi Di Malaysia, berdasarkan persetujuan, istilah ini berarti seluruh orang Melayu adalah Bumiputra dan seluruh Bumiputra adalah orang Melayu. Namun istilah ini tidak benar secara tek...
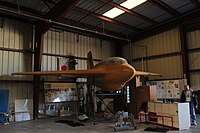
Mitsubishi J8M Shusui (Jepang: 三菱 J8M 秋水, harfiah Autumn Water, digunakan sebagai istilah puitis yang berarti Sharp Sword berasal dari pedang suara mendesir membuat) adalah pesawat pencegat pada Perang Dunia II bertenaga roket milik Jepang yang berbasis erat dengan Messerschmitt Me 163 Komet miik Jerman. Dibangun sebagai sebuah proyek bersama untuk kedua Angkatan Laut dan Udara, dan Angkatan Darat, itu ditetapkan J8M (Navy) dan Ki-200 (Army). Meskipun tidak memiliki model fungsional ...

Untuk kegunaan lain, lihat MRT. MRT JakartaInfoPemilikPemerintah Provinsi DKI JakartaWilayahJakarta, IndonesiaJenisAngkutan cepatJumlah jalur1Jumlah stasiun13 beroperasiPenumpang harian71.827 (Desember 2022)[1]Pimpinan utamaTuhiyatKantor pusatKompleks Wisma Nusantara lantai 21Jalan M.H. Thamrin No. 59Kelurahan Gondangdia, Kecamatan MentengJakarta Pusat 10350Situs webwww.jakartamrt.co.idOperasiDimulai24 Maret 2019; 5 tahun lalu (2019-03-24)OperatorPT Mass Rapid Transit Jakarta (Pe...
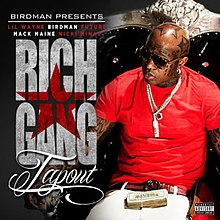
Not to be confused with Tap Out (song). 2013 single by Rich Gang featuring Birdman, Lil Wayne, Mack Maine, Nicki Minaj and FutureTapoutSingle by Rich Gang featuring Birdman, Lil Wayne, Mack Maine, Nicki Minaj and Futurefrom the album Rich Gang B-sideFly RichReleasedMarch 12, 2013Recorded2012–2013Genre Hip hop dirty rap trap Length4:44LabelCash MoneyYoung MoneyRepublicSongwriter(s) Dwayne Carter Nayvadius Wilburn Noel Fisher Onika Tanya Maraj Jermaine Preyan Bryan Williams Joshua Luellen Bry...

British actor (1923–2014) The Right HonourableThe Lord AttenboroughCBE FRSAAttenborough in 1975BornRichard Samuel Attenborough(1923-08-29)29 August 1923Cambridge, EnglandDied24 August 2014(2014-08-24) (aged 90)Northwood, London, EnglandResting placeSt Mary Magdalene, Richmond, LondonOccupationsActorfilm directorproducerPolitical partyLabourSpouse Sheila Sim (m. 1945)ChildrenMichaelJaneCharlotteParentFrederick Attenborough (father)Relatives David ...

VectorLinuxDeluxe Soho Edition Desktop 5.9Perusahaan / pengembangRobert S. Lange; Darrell StavemKeluargaMirip UnixStatus terkiniCurrentModel sumberOpen sourceRilis stabil terkini7.0 GOLD[1] / 27 November 2011; 12 tahun lalu (2011-11-27)Kernel typeMonolithicAntarmuka bawaanXfce, KDE, LXDE, Openbox, JWMLisensiVariousSitus web resmiwww.vectorlinux.com VectorLinux, disingkat VL, adalah sistem operasi Linux untuk platform x86 berbasis Slackware Linux. Awalnya dikembangkan oleh pe...
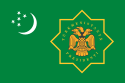
土库曼斯坦总统土库曼斯坦国徽土库曼斯坦总统旗現任谢尔达尔·别尔德穆哈梅多夫自2022年3月19日官邸阿什哈巴德总统府(Oguzkhan Presidential Palace)機關所在地阿什哈巴德任命者直接选举任期7年,可连选连任首任萨帕尔穆拉特·尼亚佐夫设立1991年10月27日 土库曼斯坦土库曼斯坦政府与政治 国家政府 土库曼斯坦宪法 国旗 国徽 国歌 立法機關(英语:National Council of Turkmenistan) ...

Portuguese singer (born 1987) AureaAurea in 2022Background informationBirth nameAurea SousaBorn (1987-09-07) 7 September 1987 (age 36)Santiago do Cacém, PortugalOriginAlvalade Do Sado, PortugalGenresSoul, blues, pop, reggae, R&B, Blue-eyed soulOccupation(s)SingerInstrument(s)VocalYears active2008–presentLabelsBlim Records / Sony MusicMusical artist Áurea Isabel Ramos de Sousa (born 7 September 1987), known professionally as Aurea, is a Portuguese soul singer originally from Santi...

For other Pennsylvania townships of the same name, see Elk Township, Pennsylvania. For other Pennsylvania townships with similar names, see Elk Creek Township, Erie County, Pennsylvania; Elk Lick Township, Somerset County, Pennsylvania; and Elkland Township, Sullivan County, Pennsylvania. Township in Pennsylvania, United StatesElk TownshipTownshipGlen Hope Covered BridgeLocation of Elk Township in Chester County (left) and of Chester County in Pennsylvania (right)Coordinates: 39°43′30″N ...

Athabaskan language of North America KoyukonDenaakkenaageʼ, Denaakkʼe, Dinaak̲'aPronunciation[təˈnæːqʼə]Native toUnited StatesRegionAlaska (middle Yukon River, Koyukuk River)EthnicityKoyukonNative speakers65 (2015 census)[1]Language familyDené–Yeniseian? Na-DenéAthabaskanNorthern AthabaskanKoyukonWriting systemLatinOfficial statusOfficial language in Alaska[2]Language codesISO 639-3koyGlottologkoyu1237ELPKoyukonKoyukon is classified as Critical...

Canadian filmmaker (born 1985) Kazik RadwanskiRadwanski at Berlinale 2020 presenting his film Anne at 13.000 ftBorn1985 (age 38–39)CanadaOccupation(s)Director, writer, producerYears active2007–present Kazik Radwanski is a Canadian film director and screenwriter. His early short films have been cited as part of the New Canadian Cinema movement.[1][2] He made his feature film directorial debut in 2012 with Tower.[3] His second feature film, How Heavy Th...

Nama ini menggunakan cara penamaan Spanyol: nama keluarga pertama atau paternalnya adalah Aedo dan nama keluarga kedua atau maternalnya adalah Santana. Daniela AedoNama asalDaniela Aedo SantanaLahir12 Februari 1995 (umur 29)Kota Meksiko, MeksikoKebangsaan MeksikoPekerjaanAktrisTahun aktif1998–sekarangOrang tuaJosé Antonio Aedo (ayah) dan Irma Santana (ibu)[1] Daniela Aedo Santana (lahir 12 Februari 1995) adalah seorang aktris berkebangsaan Meksiko. Ia...

Jacques Marquette, pioneer missionary to Native Americans The Jesuits in the United States constitute the American branch of the Society of Jesus and are organized into four geographic provinces — East, Central and Southern, Midwest and West — each of which is headed by a provincial superior. The order is known, historically, for its missions to the Native Americans in the early 17th century, for owning and participating in the Atlantic slave trade, and, contemporarily, for its network o...

Maureen O'Sullivan nel 1932 Maureen Paula O'Sullivan (Boyle, 17 maggio 1911 – Scottsdale, 23 giugno 1998) è stata un'attrice irlandese naturalizzata statunitense. Indice 1 Biografia 2 Filmografia parziale 2.1 Cinema 2.2 Televisione 3 Doppiatrici italiane 4 Note 5 Voci correlate 6 Altri progetti 7 Collegamenti esterni Biografia Maureen O'Sullivan ebbe una delle carriere più lunghe della storia di Hollywood, durata dal 1930 al 1994. Nata a Boyle, nella contea irlandese di Roscommon, studiò...
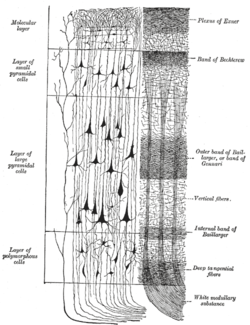
Mammalian structure involved in higher-order brain functions For other uses, see Neocortex (disambiguation). NeocortexA representative column of neocortex. Cell body layers are labeled on the left, and fiber layers are labeled on the right.IdentifiersMeSHD019579NeuroNames757NeuroLex IDbirnlex_2547TA98A14.1.09.304 A14.1.09.307TA25532FMA62429Anatomical terms of neuroanatomy[edit on Wikidata] The neocortex, also called the neopallium, isocortex, or the six-layered cortex, is a set of layers ...