Jacobi method
|
Read other articles:
Kejuaraan Dunia U-17 FIFA 1993(Jepang) 1993 FIFA U-17世界選手権Logo Kejuaraan Dunia U-17 FIFA 1993Informasi turnamenTuan rumahJepangJadwalpenyelenggaraan21 Agustus – 4 September 1993Jumlahtim peserta16 (dari 6 konfederasi)Tempatpenyelenggaraan6 (di 6 kota)Hasil turnamenJuara Nigeria (gelar ke-2)Tempat kedua GhanaTempat ketiga ChiliTempat keempat PolandiaStatistik turnamenJumlahpertandingan32Jumlah gol107 (3,34 per pertandingan)Jumlahpenonton233.004...

Moshe ben Maimon (Maimonides)Lahir1135Córdoba, Spanyol, Al-Murabithun (sekarang Spanyol)Meninggal12 Desember 1204 (usia 69)Fostat, Mesir, atau Cairo, Mesir[1]EraFilsafat Abad PertengahanKawasanArab MediteraniaAliranFilsafat Yahudi, Hukum Yudaisme, Etika Yudaisme Dipengaruhi Talmud, Aristoteles, al-Farabi, Ibnu Sina, Ibnu Bajjah, Ar-Razi, Al-Ghazali[2][3] Memengaruhi Jeremiah Stamler, Spinoza, Aquinas, Joyce, Bodin, Leibniz, Newton,[4] Strauss, Friedländer, L...
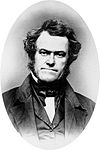
Maine's 5th congressional districtObsolete districtCreated1821Eliminated1883Years active1821-1883 Maine's 5th congressional district was a congressional district in Maine. It was created in 1821 after Maine achieved statehood in 1820. It was eliminated in 1883. Its last congressman was Thompson Henry Murch. List of members representing the district Member Party Years ↑ Congress Electoral history District location District created March 4, 1821 Ebenezer Herrick(Bowdoinham) Democratic-Republ...

العلاقات الجزائرية الليسوتوية الجزائر ليسوتو الجزائر ليسوتو تعديل مصدري - تعديل العلاقات الجزائرية الليسوتوية هي العلاقات الثنائية التي تجمع بين الجزائر وليسوتو.[1][2][3][4][5] مقارنة بين البلدين هذه مقارنة عامة ومرجعية للدولتين: وجه الم...

العلاقات الغرينادية الكوستاريكية غرينادا كوستاريكا غرينادا كوستاريكا تعديل مصدري - تعديل العلاقات الغرينادية الكوستاريكية هي العلاقات الثنائية التي تجمع بين غرينادا وكوستاريكا.[1][2][3][4][5] مقارنة بين البلدين هذه مقارنة عامة ومرجعي�...

Dewan Perwakilan Rakyat Daerah Kabupaten BojonegoroDewan Perwakilan RakyatKabupaten Bojonegoro2019-2024JenisJenisUnikameral Jangka waktu5 tahunSejarahSesi baru dimulai21 Agustus 2019PimpinanKetuaAbdullah Umar, S.Pd. (PKB) sejak 2 Februari 2022 Wakil Ketua IH. Sukur Priyanto, S.E., M.A.P. (Demokrat) sejak 17 September 2019 Wakil Ketua IISahudi, S.E. (Gerindra) sejak 17 September 2019 Wakil Ketua IIIHj. Mitro’atin, S.Pd. (Golkar) sejak 17 September 2019 KomposisiAnggota50Parta...

International airport in Varanasi, Uttar Pradesh, India Lal Bahadur Shastri International Airport IATA: VNSICAO: VEBNSummaryAirport typePublicOwnerGovernment of IndiaOperatorAirports Authority of IndiaServesVaranasiLocationBabatpur, Varanasi district, Uttar Pradesh, IndiaElevation AMSL270 ft / 82.30 mCoordinates25°27′08″N 082°51′34″E / 25.45222°N 82.85944°E / 25.45222; 82.85944WebsiteVaranasi AirportMapVNSLocation of airport in Uttar PradeshS...

Artikel ini sebatang kara, artinya tidak ada artikel lain yang memiliki pranala balik ke halaman ini.Bantulah menambah pranala ke artikel ini dari artikel yang berhubungan atau coba peralatan pencari pranala.Tag ini diberikan pada Oktober 2022. Nanokapasitor adalah kapasitor dengan ukuran nanometer. Bahan pembuatannya adalah logam yang berbentuk bola. Nilai kapasitansi nanokapasitor sebesar 1,11 × 10−19 Farad. Pada kondisi normal, nanokapasitor tidak bermuatan listrik. Nanokapasitor hanya ...
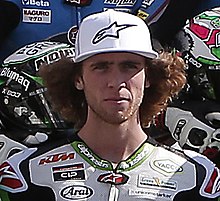
Darryn BinderBinder di tahun 2019KebangsaanAfrika SelatanLahir21 Januari 1998 (umur 26)Potchefstroom, Afrika SelatanTim saat iniPetronas Sprinta RacingNo. motor40 Catatan statistik Karier Kejuaraan Dunia Moto3Tahun aktif2015– PabrikanMahindra, KTM Klasemen 20208th (122 poin) Start Menang Podium Pole F. lap Poin 100 1 5 2 4 311 Darryn Binder (lahir 21 Januari 1998) adalah pembalap motor profesional Afrika Selatan yang berkompetisi di kelas Moto2. Karier Binder lahir di Potchefstroom, Af...

Neil ArmstrongUnited States Navy/Astronauta della NASANazionalità Stati Uniti StatusDeceduto Data di nascita5 agosto 1930 Data di morte25 agosto 2012 Selezione1962 (gruppo 2 NASA) Primo lancio16 marzo 1966 Ultimo atterraggio24 luglio 1969 Altre attivitàPilota collaudatore Tempo nello spazio8 giorni, 14 ore, 12 minuti e 30 secondi Numero EVA1 Durata EVA2 h 31 min Missioni Gemini 8 Apollo 11 Data ritiroagosto 1970 Modifica dati su Wikidata · Manuale (EN) «That's one small step for...

Form of entropy encoding used in data compression This article includes a list of general references, but it lacks sufficient corresponding inline citations. Please help to improve this article by introducing more precise citations. (September 2016) (Learn how and when to remove this message) Arithmetic coding (AC) is a form of entropy encoding used in lossless data compression. Normally, a string of characters is represented using a fixed number of bits per character, as in the ASCII code. W...

تيست درايف سيكلز Test Drive Cycles غلاف اللعبة عبر جيم بوي كولر المطور زانتيرا الناشر إنفوجرامز أمريكا الشمالية سلسلة اللعبة تيست درايف النظام جيم بوي تاریخ الإصدار أغسطس 2000 (أ.ش) نوع اللعبة لعبة سباق الدراجات النارية النمط لعبة فيديو فرديةلعبة فيديو جماعية الوسائط تحميل التقييم...

Bulgarian footballer Vladislav Zlatinov Zlatinov playing for Beroe in 2010Personal informationFull name Vladislav Hristov ZlatinovDate of birth (1983-03-23) 23 March 1983 (age 41)Place of birth Sandanski, BulgariaHeight 1.82 m (6 ft 0 in)Position(s) ForwardTeam informationCurrent team Septemvri SimitliNumber 9Youth career Vihren CSKA SofiaSenior career*Years Team Apps (Gls)2002–2003 Vihren 19 (7)2003–2005 Pirin Blagoevgrad 53 (35)2005–2008 Lokomotiv Plovdiv 58 (10)20...

This biography of a living person needs additional citations for verification. Please help by adding reliable sources. Contentious material about living persons that is unsourced or poorly sourced must be removed immediately from the article and its talk page, especially if potentially libelous.Find sources: Marie Ortal Malka – news · newspapers · books · scholar · JSTOR (May 2010) (Learn how and when to remove this message) Marie Ortal Malka (born 13 ...

This article needs additional citations for verification. Please help improve this article by adding citations to reliable sources. Unsourced material may be challenged and removed.Find sources: Pakistanis in Oman – news · newspapers · books · scholar · JSTOR (January 2021) (Learn how and when to remove this message) Ethnic group Pakistanis in OmanA Pakistani restaurant in RustaqTotal population231,685 (November 2016)[1]Regions with significant...

Bagian dari seriGereja Katolik menurut negara Afrika Afrika Selatan Afrika Tengah Aljazair Angola Benin Botswana Burkina Faso Burundi Chad Eritrea Eswatini Etiopia Gabon Gambia Ghana Guinea Guinea-Bissau Guinea Khatulistiwa Jibuti Kamerun Kenya Komoro Lesotho Liberia Libya Madagaskar Malawi Mali Maroko Mauritania Mauritius Mesir Mozambik Namibia Niger Nigeria Pantai Gading Republik Demokratik Kongo Republik Kongo Rwanda Sao Tome dan Principe Senegal Seychelles Sierra Leone Somalia Somaliland ...
City in Kantō, JapanKatori 香取市City Sawara old townKatori JinguSawara Mitsubishi-kanSawara O-MatsuriIno Tadataka house Suigō Sawara Iris Park FlagSealLocation of Katori in Chiba PrefectureKatori Coordinates: 35°41′N 140°2′E / 35.683°N 140.033°E / 35.683; 140.033CountryJapanRegionKantōPrefectureChibaGovernment • MayorSeiichi Ui (since May 2006)Area • Total262.31 km2 (101.28 sq mi)Population (November 1, 20...

Noah O. KnightPenerima Medal of HonorLahir(1929-10-27)27 Oktober 1929McBee, Carolina SelatanMeninggal24 November 1951(1951-11-24) (umur 22)dekat Kowang-San, KoreaTempat pemakamanUnion Hill Baptist Church Cemetery, Pageland, Carolina SelatanPengabdianAmerika SerikatDinas/cabangAngkatan Darat Amerika SerikatLama dinas-1951PangkatPrivat Kelas SatuKesatuanCompany F, 7th Infantry Regiment, 3rd Infantry DivisionPerang/pertempuranPerang Korea (DOW)PenghargaanMedal of HonorPurple Hear...

У Вікіпедії є статті про інші значення цього терміна: Ягода (значення). Чотири типи дійсних ягід; за годинниковою стрілкою справа: виноград, хурма, аґрус, порічки (верх). Кілька типів «ягід» у звичайному розумінні, жодна з яких не є ягодою з ботанічної точки зору; згори дон...

Railway station in Northern Ireland This article needs additional citations for verification. Please help improve this article by adding citations to reliable sources. Unsourced material may be challenged and removed.Find sources: Adavoyle railway station – news · newspapers · books · scholar · JSTOR (November 2015) (Learn how and when to remove this message) AdavoyleRemains of the station photographed on 24 August 2007General informationLocationAdavoy...