عودية (علم الحاسوب)
|
Read other articles:

New Found GloryAsalCoral Springs, Florida, Amerika SerikatGenrePop punkHardcore[1][2][3][4]Tahun aktif1997 – sekarangLabelDrive-Thru, Geffen, Bridge 9Artis terkaitShai HuludThe International Superheroes of HardcoreSitus webSitus ResmiAnggotaJordan PundikChad GilbertSteve KleinIan GrushkaCyrus BolookiMantan anggotaJoe Moreno New Found Glory merupakan sebuah grup musik yang berasal dari Coral Springs, Florida, Amerika Serikat. Band ini didirikan pada tahun 1997...

If Cats Disappeared from the WorldPosterNama lainJepang世界から猫が消えたならHepburnSekai kara Neko ga Kieta nara SutradaraAkira Nagai [ja]BerdasarkanSekai kara Neko ga Kieta nara [ja]oleh Genki Kawamura [ja]Pemeran Takeru Satoh Aoi Miyazaki Takeru Satoh[1] Aoi Miyazaki[1] Gaku Hamada[1] Eita Okuno [ja][1] Anna Ishii[1] Eiji Okuda[1] Mieko Harada[1] DistributorTohoTangg...

American mathematician and educator Andrew M. GleasonBerlin, 1959Born(1921-11-04)November 4, 1921Fresno, CaliforniaDiedOctober 17, 2008(2008-10-17) (aged 86)Cambridge, MassachusettsAlma materYale University[3]Known for Hilbert's fifth problem Gleason's theorem Greenwood–Gleason graph Gleason–Prange theorem Gleason polynomials Spouse Jean Berko Gleason (m. 1959)Awards Newcomb Cleveland Prize (1952) Gung–Hu Distinguished Servic...

Number in base-10 numeral system For other uses, see Decimal (disambiguation). Place value of number in decimal system The decimal numeral system (also called the base-ten positional numeral system and denary /ˈdiːnəri/[1] or decanary) is the standard system for denoting integer and non-integer numbers. It is the extension to non-integer numbers (decimal fractions) of the Hindu–Arabic numeral system. The way of denoting numbers in the decimal system is often referred to as decima...
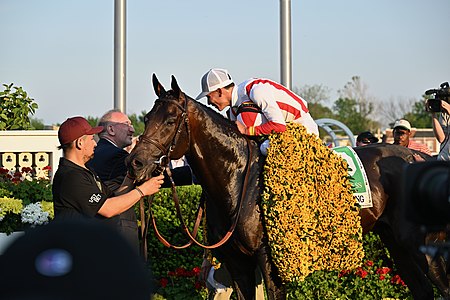
American-bred Thoroughbred racehorse This article is about the American Thoroughbred racehorse. For the voting process, see Early voting. Early VotingEarly Voting at Preakness StakesSireGun RunnerGrandsireCandy Ride (ARG)DamAmour D'EteDamsireTiznowSexColtFoaledMarch 7, 2019[1]CountryUnited StatesColourBayBreederThree Chimneys FarmOwnerKlaravich StablesTrainerChad C. BrownRecord6: 3-1-0Earnings$1,372,500[2]Major winsWithers Stakes (2022)American Triple Crown wins:Preakness Stak...

この項目には、一部のコンピュータや閲覧ソフトで表示できない文字が含まれています(詳細)。 数字の大字(だいじ)は、漢数字の一種。通常用いる単純な字形の漢数字(小字)の代わりに同じ音の別の漢字を用いるものである。 概要 壱万円日本銀行券(「壱」が大字) 弐千円日本銀行券(「弐」が大字) 漢数字には「一」「二」「三」と続く小字と、「壱」「�...

此條目可参照英語維基百科相應條目来扩充。 (2021年5月6日)若您熟悉来源语言和主题,请协助参考外语维基百科扩充条目。请勿直接提交机械翻译,也不要翻译不可靠、低品质内容。依版权协议,译文需在编辑摘要注明来源,或于讨论页顶部标记{{Translated page}}标签。 约翰斯顿环礁Kalama Atoll 美國本土外小島嶼 Johnston Atoll 旗幟颂歌:《星條旗》The Star-Spangled Banner約翰斯頓環礁�...

2016年美國總統選舉 ← 2012 2016年11月8日 2020 → 538個選舉人團席位獲勝需270票民意調查投票率55.7%[1][2] ▲ 0.8 % 获提名人 唐納·川普 希拉莉·克林頓 政党 共和黨 民主党 家鄉州 紐約州 紐約州 竞选搭档 迈克·彭斯 蒂姆·凱恩 选举人票 304[3][4][註 1] 227[5] 胜出州/省 30 + 緬-2 20 + DC 民選得票 62,984,828[6] 65,853,514[6]...

الاشتباك الجوي السعودي الإيراني جزء من حرب الخليج الأولى طائرة إف 15 سعودية معلومات عامة التاريخ 5 يونيو 1984 البلد السعودية الموقع الخليج العربي، 60 ميل من شمال شرق الجبيل، السعودية النتيجة إسقاط طائرتين إيرانيتين و إصابة ثالثة انتصار سعودي حاسم المتحاربون إيران ا...

Adenosine diphosphate ribose Names Other names ADP riboseADPRAdenosine 5'-diphosphoribose Identifiers CAS Number 20762-30-5 N 3D model (JSmol) Interactive image ChEMBL ChEMBL1161865 N ChemSpider 388340 N MeSH Adenosine+Diphosphate+Ribose PubChem CID 439200 UNII XV3S4KV26E Y InChI InChI=1S/C15H23N5O14P2/c16-12-7-13(18-3-17-12)20(4-19-7)14-10(24)9(23)6(31-14)2-30-35(26,27)34-36(28,29)33-15-11(25)8(22)5(1-21)32-15/h3-6,8-11,14-15,21-25H,1-2H2,(H4,16,17,18,26,27,28,29)/p+1/t5...
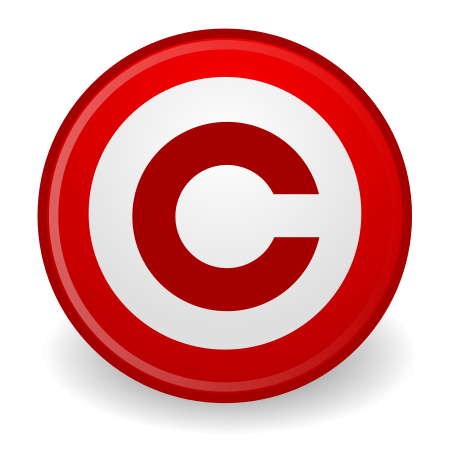
ملخص معلومات الملف الوصف هذه صورة لشخصية: حسن كامي المصدر يوتيوب التاريخ أبريل 2018 المنتج DMC الإذن(إعادة الاستخدام) انظر أدناه ترخيص هذه صورةٌ لشخصية متوفاة وهي محميةٌ بحقوق التأليف والنشر. في ويكيبيديا يسمح برفع واستخدام صور للشخصيات المتوفاة تحت الاستعمال العادل وفق قانو...
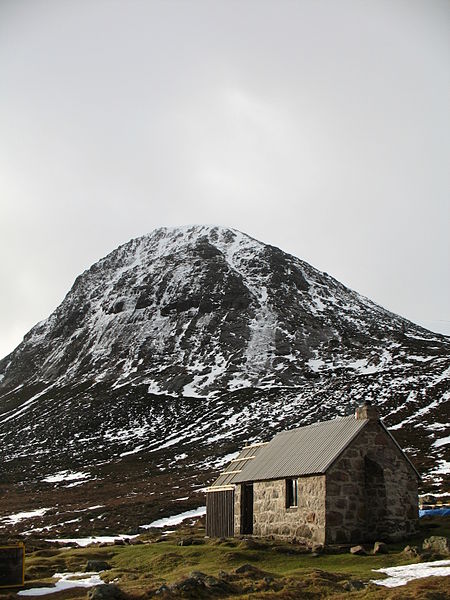
Not to be confused with Devil's Point, Devon. The Devil's PointBod an DeamhainHighest pointElevation1,004 m (3,294 ft)[1]Prominencec. 89 mParent peakCairn ToulListingMunroNamingEnglish translationPenis of the demonLanguage of nameScottish GaelicPronunciationScottish Gaelic: [ˈpɔt̪ əɲ ˈtʲãũ.ɪɲ]GeographyLocationCairngorms, ScotlandOS gridNN976951Topo mapOS Landranger 43 The Devil's Point (Scottish Gaelic: Bod an Deamhain) is a mountain in the Cairngorms...

Indian politician and Minister This biography of a living person needs additional citations for verification. Please help by adding reliable sources. Contentious material about living persons that is unsourced or poorly sourced must be removed immediately from the article and its talk page, especially if potentially libelous.Find sources: Darshana Jardosh – news · newspapers · books · scholar · JSTOR (July 2021) (Learn how and when to remove this messa...
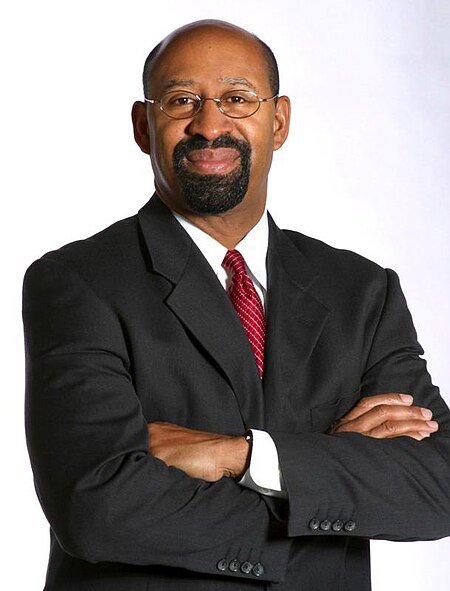
Mayor of Philadelphia from 2008 to 2016 This article has multiple issues. Please help improve it or discuss these issues on the talk page. (Learn how and when to remove these template messages) This article contains content that is written like an advertisement. Please help improve it by removing promotional content and inappropriate external links, and by adding encyclopedic content written from a neutral point of view. (February 2016) (Learn how and when to remove this message) This biograp...
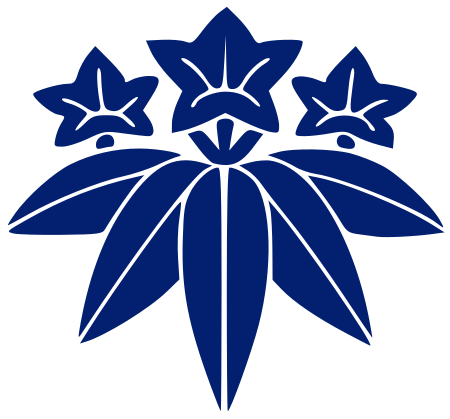
Perang Genpei (Perang Gempei)Bagian dari Persengketaan klan Minamoto–Taira pada akhir zaman HeianAdegan perang GenpeiTanggal1180–1185LokasiJepangHasil Kemenangan klan Minamoto; Keshogunan Kamakura didirikanPihak terlibat klan Minamoto (Yoritomo) klan Taira klan Minamoto (Yoshinaka)Tokoh dan pemimpin Minamoto no Yoritomo, Minamoto no Yoshitsune Taira no Munemori , Taira no Shigehira , Taira no Tomomori † Minamoto no Yoshinaka †, Imai Kanehira †Korban 20000 30...

2001 studio album by Uri CaineRioStudio album by Uri CaineReleased2001RecordedJune 8–13, 2001 Various locations in Rio de Janeiro, BrazilGenreJazzLength69:06LabelWinter & Winter 910 079ProducerUri Caine & Stefan WinterUri Caine chronology Solitaire(2001) Rio(2001) Diabelli Variations(2002) Rio is an album by Uri Caine which was recorded and released on the Winter & Winter label in 2001.[1][2] The album explores Brazilian music, and features of large ensem...
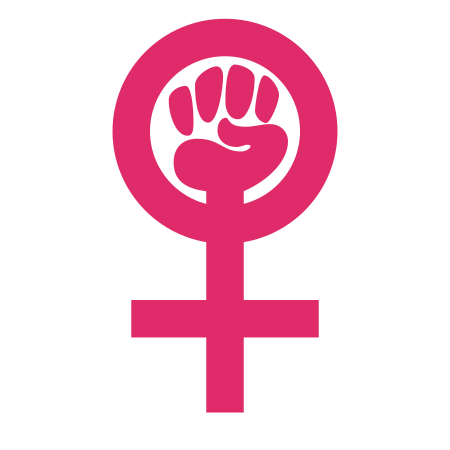
Une cabine téléphonique de Londres Un échange économico-sexuel est une transaction économique liée à l’exécution (ou la promesse) d'un acte sexuel. Ce concept du matérialisme historique est utilisé en sciences sociales depuis sa formalisation à la fin du XXe siècle par l'anthropologue Italienne Paola Tabet. Pour elle, bien que le « sens commun » dissocie en Europe la prostitution des autres formes de relations sexuelles, celles-ci sont aussi marquées par la pr�...

此條目介紹的是中国福建省漳平市漳平站至广东省龙川县龙川站的铁路。关于本线的一部分,原漳平至龙岩铁路,请见「漳龙铁路 (漳平至龙岩)」。关于客运运价里程表记载的龙漳线(龙岩-漳州铁路),请见「龙厦铁路」。 漳龙铁路漳龙线漳平段概覽运营範圍中国福建省、广东省服務類型客货两用目前狀況使用中起點站漳平站-龙川站技術數據线路長度374千米正線...

Ynyscedwyn IronworksRemains of the unfinished structures at Ynyscedwyn Ironworks, begun in 1872Established1612 (412 years ago)Dissolved1978 Typesironworks Coordinates51°46′06″N 3°45′51″W / 51.768365°N 3.764263°W / 51.768365; -3.764263 Ynyscedwyn Ironworks is an industrial complex located in Ystradgynlais, near Swansea, Wales. Smelting was first established here in seventeenth century. In the 1820s, with the arrival of George Crane, prod...

Atletik - 200 meter putri pada Paralimpiade Musim Panas 2024LokasiStade de FranceTanggal30 August – 7 September 2024Jumlah disiplin7 200m Putri atletik pada Paralimpiade Musim Panas 2024 akan berlangsung di Stade de France dari 30 Agustus hingga 7 September 2024. Total ada 7 nomor yang akan diperlombakan pada jarak ini. Jadwal R Babak 1 ½ Semifinal F Final Tanggal[1] Jum 30 Sab 31 Min 1 Sen 2 Sel 3 Rab 4 Kam 5 Jum 6 Sab 7 Event P M P M P M P M P M P M P M P M P M 200m T11 R ½ F 20...