X86调用约定
|
Read other articles:
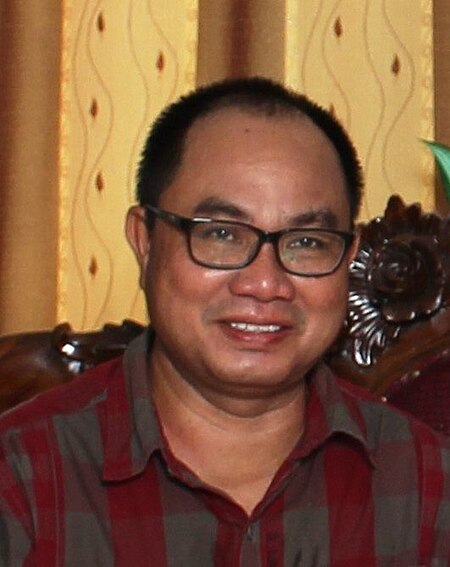
Jhon Retei Alfri SandiLahir12 April 1970 (umur 53)Kuala Kapuas, Kalimantan Tengah, IndonesiaPekerjaanDosen, AkademisiOrganisasiUniversitas Palangka RayaSuami/istriTelhalia, M.Th., D.Th.Anak4 Dr. Jhon Retei Alfri Sandi, S.Sos., M.Si. (lahir 12 April 1970) adalah seorang dosen, akademisi, pengamat politik, dan tokoh pendidikan berkebangsaan Indonesia yang berkiprah di Kalimantan Tengah. Kehidupan awal Jhon Retei Alfri Sandi dilahirkan di Kuala Kapuas, Kalimantan Tengah, Indonesia. Ia anak...

Film komedi romantis atau romansa komedi (Inggris: romantic comedycode: en is deprecated , romcom) adalah film percintaan dengan alur cerita yang riang dan jenaka, berpusat pada kondisi ideal romantis, seperti misalnya kisah cinta sejati yang dapat mengatasi berbagai hambatan. Film komedi romantis adalah sub-genre film komedi dan film romantis. Contoh film yang termasuk genre ini adalah Valentine's Day (2010). Komedi romantis juga dapat digunakan untuk menggambarkan beberapa serial televisi. ...

Artikel ini sebatang kara, artinya tidak ada artikel lain yang memiliki pranala balik ke halaman ini.Bantulah menambah pranala ke artikel ini dari artikel yang berhubungan atau coba peralatan pencari pranala.Tag ini diberikan pada Desember 2023. Recep TopalInformasi pribadiLahir29 Oktober 1992 (umur 31)Tinggi169 cm (5,54 ft; 67 in) OlahragaNegaraTurkiOlahragaGulat amatirKelas berat61 kgLombaGaya bebas Rekam medali Gulat gaya bebas putra Mewakili Turki Kejuaraan ...
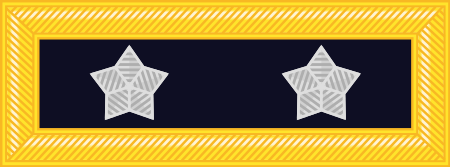
Untuk senator negara bagian Oregon, lihat Lew Wallace (1889-1960). Lew Wallace Gubernur Teritorial New Mexico ke-11Masa jabatan1878–1881 PendahuluSamuel Beach AxtellPenggantiLionel Allen SheldonMenteri Amerika Serikat untuk Kekaisaran UtsmaniyahMasa jabatan1881–1885 PendahuluJames LongstreetPenggantiSamuel S. Cox Informasi pribadiLahirLewis Wallace10 April 1827 (1827-04-10)Brookville, IndianaMeninggalNot recognized as a date. Years must have 4 digits (use leading zeros for years <...

Ini adalah nama Minahasa, marganya adalah Lumintang Johny LumintangJohny Lumintang Duta Besar Indonesia untuk Filipina Masa jabatan14 Februari 2014 – 20 Februari 2018 PendahuluYohanes K. LegowoPenggantiSinyo Harry SarundajangGubernur Lemhannas ke-12Masa jabatan1999–2001 PendahuluAgum GumelarPenggantiErmaya Suradinata Informasi pribadiLahir28 Juni 1947 (umur 76)Noongan, Langowan Barat, Minahasa, SulawesiSuami/istridrg. Sonya Riupassa, M.H.A.AnakKitty LumintangDella Lumint...

Robert PattinsonRobert Pattinson, pada tahun 2017LahirRobert Thomas Pattinson13 Mei 1986 (umur 37)London, InggrisPekerjaanAktor, modelTahun aktif2004—sekarangSitus webRobertpattinson.comPattinson saat premier Twilight di Los Angeles Robert Douglas Thomas Pattinson[1][2] (lahir 13 Mei 1986)[3] adalah pemeran, model dan musisi Inggris,[4] terkenal dalam perannya sebagai Cedric Diggory dalam film Harry Potter and the Goblet of Fire.[5] Ia berm...
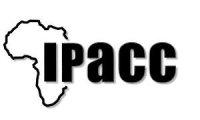
Main trans-national network organizationsIPACC logo Part of a series onIndigenous rights Rights Ancestral domain Intellectual property Land rights Language Traditional knowledge Treaty rights Water and sanitation Protection Governmental organizations ACHPR AID Arctic Council BIA CIP CIRNAC DTA FUNAI INPI JAKOA NCIP NIAA MCHTA TPK UNPFII NGOs and political groups AFN Amazon Conservation Team Amazon Watch CAP COICA CONAECDA CONAIE Cultural Survival EZLN fPcN IPACC IPCB IWGIA Land Back NARF ONIC...

Shriniwas Dadasaheb PatilShriniwas Dadasaheb Patil Gubernur SikkimPetahanaMulai menjabat 1 Juli 2013PendahuluBalmiki Prasad SinghPenggantiPetahanaAnggota Parlemen India untuk KaradMasa jabatan1999–2009PendahuluPrithviraj ChavanPenggantiPetahana Informasi pribadiLahir11 April 1941 (umur 83)Satara, MaharashtraPartai politikNCPSuami/istriRajanideviAnak2 putraTempat tinggalSataraPer tanggal 16 September, 2006Sumber: [1]Sunting kotak info • L • B Shriniwas Dadasaheb Patil ...

Questa voce sull'argomento stagioni delle società calcistiche italiane è solo un abbozzo. Contribuisci a migliorarla secondo le convenzioni di Wikipedia. Segui i suggerimenti del progetto di riferimento. Voce principale: Nazionale Lombardia Foot-Ball Club. S.C. Italia S.C. Nazionale LombardiaStagione 1919-1920Sport calcio Squadra Nazionale Lombardia Allenatore Commissione Tecnica Presidente Giovanni Sfondrini[1] Prima Categoria5º nel girone C. 1918-1919 1920-1921 Si...

Friedhof St. Magdalena Der Friedhof St. Magdalena zählt zur Gruppe der Friedhöfe in Linz und liegt im Stadtteil St. Magdalena. Der Friedhof wird von der Pfarre St. Magdalena betrieben. Inhaltsverzeichnis 1 Geschichte 2 Gräber 3 Galerie 4 Weblinks Geschichte Der Friedhof ist seit dem 17. Jahrhundert erwähnt und befand sich wie in allen Dörfern Oberösterreichs um die Pfarrkirche. 1835 wurde der Friedhof an den heutigen Standort an der Oberbairinger Straße verlegt und wurde im Lauf der Z...

Association football club in England Football clubPadihamFull namePadiham Football ClubNickname(s)The StorksFounded1878; 146 years ago (1878)GroundArbories Memorial Sports Ground, PadihamCapacity1,688ChairmanShaun AstinManagerMichael MorrisonLeagueNorth West Counties League Premier Division2022–23North West Counties League Premier Division, 14th of 22 Home colours Away colours Padiham Football Club are an English football team based in Padiham, Lancashire. As of 2019–20,...

Ezgjan Alioski Alioski in azione con la Macedonia del Nord nel 2014 Nazionalità Macedonia del Nord Altezza 172 cm Peso 69 kg Calcio Ruolo Centrocampista, difensore Squadra Al-Ahli CarrieraGiovanili ????-2005 Flamatt2005-2013 Young BoysSquadre di club1 2013-2016 Sciaffusa87 (4)2016-2017 Lugano50 (19)2017-2021 Leeds Utd161 (21)2021-2022 Al-Ahli28 (6)2022-2023→ Fenerbahçe17 (1)2023- Al-Ahli4 (0)Nazionale 2009 Macedonia U-171 (0)2010-2012 ...

Enteng ng Ina MoPoster filmSutradaraTony Y. ReyesProduser Charo Santos-Concio Malou N. Santos Orlando R. Ilacad Antonio P. Tuviera Marvic Sotto Martina Eileen H. delas Alas SkenarioDanno Kristoper C. MariquitCeritaDanno Kristoper C. MariquitLawrence NicodemusPemeranVic SottoAi-Ai de las AlasPenata musikJessie LasatenSinematograferGary GardocePenyuntingCharliebebs GohetiaPerusahaanproduksi ABS-CBN Film Productions, Inc. OctoArts Films APT Entertainment M-Zet Productions DistributorStar C...

I Bolivarian GamesHost cityBogotáCountry ColombiaNations6Athletes716OpeningAugust 6, 1938 (1938-08-06)ClosingAugust 22, 1938 (1938-08-22)Opened byAlfonso López PumarejoMain venueEstadio El Campín1947/48 Lima → The I Bolivarian Games (Spanish: Juegos Bolivarianos) were a multi-sport event held between August 6–22, 1938, in Bogotá, Colombia, at the Estadio El Campín, for the city's 400th anniversary. The Games were organized by the Bolivarian...

CBS affiliate in Goldsboro, North Carolina For the radio station in New York City that held the callsign WNCN from 1957 to 1993, see WAXQ. Not to be confused with WCNC-TV, the NBC affiliate in Charlotte, North Carolina. WNCNGoldsboro–Raleigh–Durham–Fayetteville, North CarolinaUnited StatesCityGoldsboro, North CarolinaChannelsDigital: 8 (VHF)Virtual: 17BrandingCBS 17ProgrammingAffiliations17.1: CBSfor others, see § SubchannelsOwnershipOwnerNexstar Media Group(Nexstar Media Inc.)His...

Mathematical space with a notion of closeness In mathematics, a topological space is, roughly speaking, a geometrical space in which closeness is defined but cannot necessarily be measured by a numeric distance. More specifically, a topological space is a set whose elements are called points, along with an additional structure called a topology, which can be defined as a set of neighbourhoods for each point that satisfy some axioms formalizing the concept of closeness. There are several equiv...

This article does not cite any sources. Please help improve this article by adding citations to reliable sources. Unsourced material may be challenged and removed.Find sources: Matariyyah – news · newspapers · books · scholar · JSTOR (June 2009) (Learn how and when to remove this message) Al-Matariyyah or Matariye, Mataria (Arabic: المطرية), is a village in South Lebanon near the Litani river. vte Sidon District, South GovernorateCapitalSidonTow...

Yu-Gi-Oh! Arc-V is the fourth spin-off anime in the Yu-Gi-Oh! franchise and the eighth anime series overall. It is produced by Nihon Ad Systems and broadcast by TV Tokyo. It is directed by Katsumi Ono and produced by Studio Gallop. Its plot focuses on Yuya Sakaki, who is a boy seeking to become the greatest entertainer in Action Duels who brings forth a new summoning method to Duel Monsters known as Pendulum Summoning. Currently, twelve pieces of theme music are used for the series: six open...

Electoral divisions of the parliament of the United Kingdom United Kingdom parliamentary constituenciesConstituencies after the 2023 Periodic ReviewCategoryElectoral districtLocationUnited KingdomNumber650 (as of 2023)GovernmentHouse of Commons This article is part of a series onPolitics of the United Kingdom Constitution Magna Carta Bill of Rights Treaty of Union (Acts of Union) Parliamentary sovereignty Rule of law Separation of powers Other constitutional principles The Crown The Monarch (...

مقاطعة جيبسون الإحداثيات 38°19′N 87°35′W / 38.31°N 87.58°W / 38.31; -87.58 [1] تاريخ التأسيس 9 مارس 1813 سبب التسمية جون جيبسون تقسيم إداري البلد الولايات المتحدة[2] التقسيم الأعلى إنديانا العاصمة برنستون التقسيمات الإدارية برنستون خصائ...