Összetétel programtervezési minta
|
Read other articles:

Rudi Hariyansyah Wakil Bupati Pesisir Selatan ke-5Masa jabatan26 Februari 2021 – 3 November 2023BupatiRusma Yul Anwar PendahuluRusma Yul AnwarPenggantiPetahana Informasi pribadiLahir2 Juli 1982 (umur 41)Tapan, Pesisir Selatan, Sumatera Barat, IndonesiaPartai politikPANSuami/istriEliza Eka PutriAnak1Orang tuaJafri (ayah)Alma materUniversitas MH ThamrinInstitut Sains dan Teknologi NasionalSunting kotak info • L • B apt. Rudi Hariyansyah, A.Md., S.Si. (lahir 2 J...
Metro station in New Taipei, Taiwan Sanmin Senior High SchoolO53 三民高中Sanmin Senior High School station platform 1Chinese nameChinese三民高中TranscriptionsStandard MandarinHanyu PinyinSānmín GāozhōngBopomofoㄙㄢㄇㄧㄣˊ ㄍㄠ ㄓㄨㄥHakkaPha̍k-fa-sṳSâm-mìn Kô-chûngSouthern MinTâi-lôSam-bîn Koo-tiong General informationOther namesNational Open University; 空中大學LocationB1, No. 105, Sanmin Rd.Luzhou, New TaipeiTaiwanOperated byTaipei MetroLine(s) Zhonghe�...
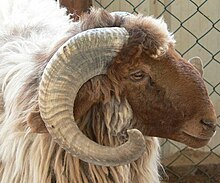
Awassi Awassi (Arab: عواسيcode: ar is deprecated ) merupakan domba lokal yang berasal dari Asia Barat Daya, lebih tepatnya berasal dari wilayah Gurun Suriah. Domba ini merupakan salah satu domba dengan ekor gemuk. Awassi memiliki nama lain Nu'aimy, ad-Deiri, as-Suri, al-Mashry, al-Baladi dan Jazirah. Awassi terdapat di negara-negara Timur Tengah, seperri Yordania, Suriah, Irak, Lebanon, Palestina dan Arab Saudi.[1] Awassi memiliki kemampuan tinggi dalam beradaptasi, dari masa ke ...

Dalam nama Spanyol ini, nama keluarganya adalah Chang. Franklin Chang DíazChang Díaz pada 1997Lahir5 April 1950 (umur 73)San José, Kosta RikaKebangsaanKosta Rika dan Amerika SerikatAlmamaterUniversitas Connecticut (BS, 1973)Massachusetts Institute of Technology (ScD, 1977)PekerjaanFisikawan, insinyur, wirausahawanKarier luar angkasaBotanis NASAWaktu di luar angkasa66 hari 18 jam 16 menitSeleksi1980 NASA GroupMisiSTS-61-C, STS-34, STS-46, STS-60, STS-75, STS-91, STS-111.Lambang misi Fr...

Hairul AzreenHairul di studio MeleTOP pada Januari 2015.LahirHairul Azreen bin Idris23 April 1988 (umur 35)Serdang, Selangor, MalaysiaPekerjaanAktor, pemeran pengganti, model, sutradara, produserTahun aktif2008–2023Tinggi178 m (584 ft 0 in)Suami/istriHanis Zalikha (m. 2015)Anak Yusuf Iskandar Alisa Aisyah Hairul Azreen Idris (lahir 23 April 1988) adalah aktor, pemeran pengganti, model dan sutradara pria Malaysia. Memulai karir di duni...

This article does not cite any sources. Please help improve this article by adding citations to reliable sources. Unsourced material may be challenged and removed.Find sources: KPWW – news · newspapers · books · scholar · JSTOR (April 2015) (Learn how and when to remove this template message) Radio station in Hooks, TexasKPWWHooks, TexasBroadcast areaTexarkana, Texas–ArkansasFrequency95.9 MHzBrandingPower 95-9ProgrammingLanguage(s)EnglishFormatTop 40...

ХристианствоБиблия Ветхий Завет Новый Завет Евангелие Десять заповедей Нагорная проповедь Апокрифы Бог, Троица Бог Отец Иисус Христос Святой Дух История христианства Апостолы Хронология христианства Раннее христианство Гностическое христианство Вселенские соборы Н...

莎拉·阿什頓-西里洛2023年8月,阿什頓-西里洛穿著軍服出生 (1977-07-09) 1977年7月9日(46歲) 美國佛羅里達州国籍 美國别名莎拉·阿什頓(Sarah Ashton)莎拉·西里洛(Sarah Cirillo)金髮女郎(Blonde)职业記者、活動家、政治活動家和候選人、軍醫活跃时期2020年—雇主內華達州共和黨候選人(2020年)《Political.tips》(2020年—)《LGBTQ國度》(2022年3月—2022年10月)烏克蘭媒�...

1920 film by Nate Watt What Women LoveAdvertisementDirected byNate WattWritten byKatherine HillikerReed HeustisVincent BryanWilliam Dudley PelleyStory byBernard McConvilleProduced bySol LesserH. P. CaulfieldStarringAnnette KellermanEdited byEdward McDermottDistributed byAssociated First NationalRelease date August 23, 1920 (1920-08-23) Running time6 reelsCountryUnited StatesLanguageSilent (English intertitles) Ralph Lewis and Annette Kellerman What Women Love is a lost[1 ...

Jon Dahl Tomasson Informasi pribadiNama lengkap Jon Dahl TomassonTanggal lahir 29 Agustus 1976 (umur 47)Tempat lahir Roskilde, DenmarkTinggi 1,82 m (5 ft 11+1⁄2 in)Posisi bermain PenyerangInformasi klubKlub saat ini FeyenoordNomor 11Karier junior1981–19851985–1992 Solrød BKKøge BKKarier senior*Tahun Tim Tampil (Gol) 1992–19941994–19971997–19981998–20022002–20052005–20072007–20082008– Køge BKSC HeerenveenNewcastle UnitedFeyenoordMilanStuttgartV...

Shooting event in South Korea 2023 Asian Shooting ChampionshipsHost city Changwon, South KoreaDates24 October – 1 November 2023Main venueChangwon Shooting Range← 20192027 → The 2023 Asian Shooting Championships were the 15th Asian Shooting Championships and took place from 22 October to 2 November 2023, at Changwon Shooting Range, Changwon, South Korea.[1][2] 813 competitors from 30 countries took part in the competition. It was the Asian qualifying tou...

Practice of the right to use a firm's business model and brand for a prescribed period of time This article is about the business concept. For other uses, see Franchise. This article has multiple issues. Please help improve it or discuss these issues on the talk page. (Learn how and when to remove these template messages) This article needs additional citations for verification. Please help improve this article by adding citations to reliable sources. Unsourced material may be challenged and ...
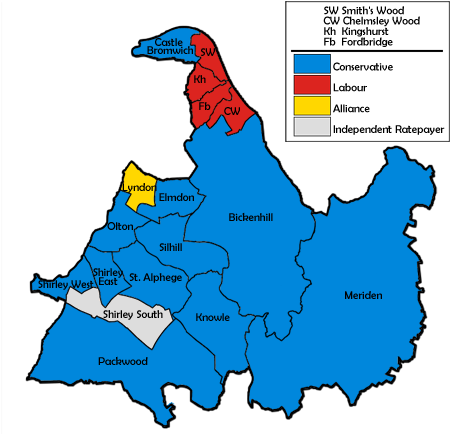
1987 UK local government election Map of the results for the 1987 Solihull council election. The 1987 Solihull Metropolitan Borough Council elections were held on Thursday, 7 May 1987, with one third of the council to be elected. The Conservatives retained control of the council. Voter turnout was 43.2%[1][2] Election result Solihull Local Election Result 1987 Party Seats Gains Losses Net gain/loss Seats % Votes % Votes +/− Conservative 11 1 1 0 64.7 51.0 34...

Artikel ini memerlukan pemutakhiran informasi. Harap perbarui artikel dengan menambahkan informasi terbaru yang tersedia. 9/11 beralih ke halaman ini. Untuk tanggal, lihat 11 September atau 9 November. Untuk kegunaan lain, lihat 911 (disambiguasi). Serangan 11 September 2001Bagian dari terorisme di Amerika SerikatDari atas, kiri ke kanan: Twin Towers yang terbakar • Para pekerja penyelamat di Ground Zero • Bagian Pentagon yang runtuh • Pecahan badan pesawat Penerbangan 93 • Kolam refl...

Period of the US Supreme Court from 1874 to 1888 Supreme Court of the United StatesWaite CourtChase Court ← → Fuller CourtChief Justice Morrison WaiteMarch 4, 1874 – March 23, 1888(14 years, 19 days)SeatOld Senate ChamberWashington, D.C.No. of positions9Waite Court decisions The Waite Court refers to the Supreme Court of the United States from 1874 to 1888, when Morrison Waite served as the seventh Chief Justice of the United States. Waite succeeded Salmon P. Chase a...

Broussy-le-Petit L'église Saint-Pierre-Saint-Paul. Administration Pays France Région Grand Est Département Marne Arrondissement Épernay Intercommunalité Communauté de communes de Sézanne-Sud Ouest Marnais Maire Mandat Nicolas Coutenceau 2020-2026 Code postal 51230 Code commune 51091 Démographie Gentilé Broussyats p'tits, Broussyates p'tites Populationmunicipale 134 hab. (2021 ) Densité 12 hab./km2 Géographie Coordonnées 48° 47′ 34″ nord, 3° 49�...

هذه المقالة يتيمة إذ تصل إليها مقالات أخرى قليلة جدًا. فضلًا، ساعد بإضافة وصلة إليها في مقالات متعلقة بها. (أبريل 2019) هيلموت فرانز معلومات شخصية تاريخ الميلاد سنة 1911 تاريخ الوفاة 30 يناير 2002 (90–91 سنة) مواطنة ألمانيا الحياة العملية المهنة قائد جوقة، ومعلم موسي�...

First women's rights convention (1848) Signers of the Declaration of Sentiments at Seneca Falls in order: Lucretia Coffin Mott is on top of the list This mahogany tea table was used on July 16, 1848, to compose much of the first draft of the Declaration of Sentiments. Part of a series onFeminism History Feminist history History of feminism Women's history American British Canadian German Waves First Second Third Fourth Timelines Women's suffrage Muslim countries US Other women's rights Women'...

Proclamation of Abdullah as leader of Transjordan, April 1921 Establishment of the Emirate of Transjordan refers to the government that was set up in Transjordan on 11 April 1921, following a brief interregnum period. Abdullah, the second son of Sharif Hussein (leader of the 1916 Great Arab Revolt against the Ottoman Empire), arrived from Hejaz by train in Ma'an in southern Transjordan on 21 November 1920. His stated aim was fighting the French in Syria, after they had defeated the short-live...

Part of a series onEcological economicsHumanity's economic system viewed as a subsystem of the global environment Concepts Carbon fee and dividend Carrying capacity Ecological market failure Ecological model of competition Ecosystem services Embodied energy Energy accounting Entropy pessimism Index of Sustainable Economic Welfare Natural capital Spaceship Earth Steady-state economy Sustainability, 'weak' vs 'strong' Uneconomic growth People Serhiy Podolynsky Frederick Soddy Nicholas Georgescu...