Three-way comparison
|
Read other articles:

Daniel Braaten Informasi pribadiNama lengkap Daniel Omoya BraatenTanggal lahir 25 Mei 1982 (umur 41)Tempat lahir Oslo, NorwegiaTinggi 1,84 m (6 ft 0 in)Posisi bermain GelandangInformasi klubKlub saat ini ToulouseNomor 25Karier junior0000–2000 SkeidKarier senior*Tahun Tim Tampil (Gol)2000–2004 Skeid 102 (22)2004–2007 Rosenborg 63 (12)2007–2008 Bolton Wanderers 6 (1)2008– Toulouse 134 (12)Tim nasional‡2003–2004 Norwegia U-21 2 (0)2004– Norwegia 42 (2) * Pena...

هذه المقالة يتيمة إذ تصل إليها مقالات أخرى قليلة جدًا. فضلًا، ساعد بإضافة وصلة إليها في مقالات متعلقة بها. (مارس 2023) كأس السوبر( الفاتيكان ) Super Coppa (Vatican City) هي كأس سنوية لمباراة كرة القدم المحلية لفرق مدينة الفاتيكان. عْقد كأس السوبر الافتتاحي عام 2005.[1] يتنافس على كأس السو�...
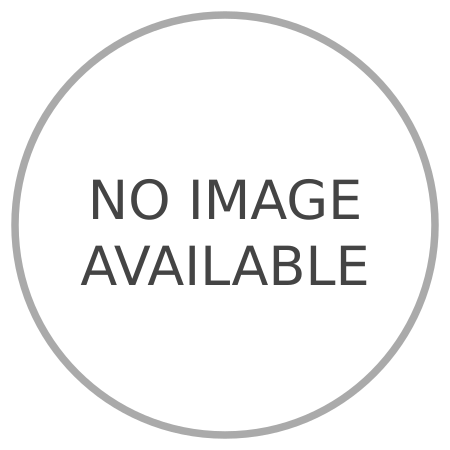
Wakil Bupati MadiunPetahanaH. Hari Wuryanto, S.H., M.Ak.sejak 24 September 2018Masa jabatan5 tahunDibentuk2003Pejabat pertamaMuhtaromSitus webmadiunkab.go.id Berikut ini adalah daftar Wakil Bupati Madiun dari masa ke masa. No Wakil Bupati Mulai Jabatan Akhir Jabatan Prd. Ket. Bupati 1 H.MuhtaromS.Sos. 2003 2008 1 H.KRH. Djunaedi MahendraS.H., M.Si. 2 Drs. H.IswantoM.Si. 23 Juli 2008 23 Juli 2013 2 H.MuhtaromS.Sos. Jabatan kosong 23 Juli 2013 3 Agustus 2013 - Soekardi(Pelaks...

Disambiguazione – Stati Uniti, USA, United States e States rimandano qui. Se stai cercando altri significati, vedi Stati Uniti (disambigua), USA (disambigua), United States (disambigua) o States (disambigua). Stati Uniti d'America (dettagli) (dettagli) In God We Trust (In Dio noi confidiamo) Stati Uniti d'America - Localizzazione Dati amministrativiNome ufficialeUnited States of America Lingue ufficialiNessuna a livello federale (de iure)[N 1] Inglese (d...

Mathias NorsgaardInformationsNom de naissance Mathias Norsgaard JørgensenNaissance 5 mai 1997 (26 ans)SilkeborgNationalité danoiseÉquipe actuelle Movistar TeamÉquipes non-UCI 2005-2011Silkeborg IF Cykling2012-2013Herning Cykle KlubÉquipes UCI 2014-2015Team Kelberg-Roskilde Junior2016SEG Racing Academy2017Giant-Castelli2018Riwal CeramicSpeed2019Riwal Readynez2020-Movistar TeamPrincipales victoires Championnats Champion du Danemark du contre-la-montre 2022modifier - modifier le code -...

Eurovision Song Contest 2004Country IsraelNational selectionSelection processArtist: Internal SelectionSong: Kdam Eurovision 2004Selection date(s)Artist: 13 November 2003Song: 5 February 2004Selected entrantDavid D'OrSelected songLeha'aminSelected songwriter(s)David D'OrOfer MeiriEhud ManorFinals performanceSemi-final resultFailed to qualify (11th)Israel in the Eurovision Song Contest ◄2003 • 2004 • 2005► Israel participated in the Eurovision Song C...

Questa voce sull'argomento stagioni delle società calcistiche italiane è solo un abbozzo. Contribuisci a migliorarla secondo le convenzioni di Wikipedia. Segui i suggerimenti del progetto di riferimento. Voce principale: Associazione Calcio Ancona. AnconitanaStagione 1945-1946Sport calcio Squadra Anconitana Allenatore Achille Piccini Divisione Nazionale11º (Centro-Sud) 1944-45 1946-47 Si invita a seguire il modello di voce Questa voce raccoglie le informazioni riguardanti la Uni...

Metropolitan Opera House di Lincoln Center for the Performing Arts, dilihat dari Lincoln Center Plaza Metropolitan Opera (the Met) adalah sebuah perusahaan opera yang terletak di New York City. Awalnya didirikan tahun 1880,[1] perusahaan ini mengadakan pertunjukan pertamanya pada 22 Oktober 1883. Perusahaan ini dioperasikan oleh asosiasi nirlaba Metropolitan Opera Association, dengan Peter Gelb sebagai manajer umumnya. Direktur musiknya ialah James Levine. The Met mengadakan pertunjuk...

Chemicals that result in blistering and skin irritation and damaging Blister agents are named for their ability to cause large, painful water blisters on the bodies of those affected. Soldier with moderate mustard gas burns sustained during World War I showing characteristic bullae on neck, armpit and hands Part of a series onChemical agents Lethal agents Blood Cyanogen chloride (CK) Hydrogen cyanide (AC) Arsine (SA) Blister Ethyldichloroarsine (ED) Methyldichloroarsine (MD) Phenyldichloroars...
1947 aviation accident Pan Am Flight 121Lockheed L-049 Constellation in PAN AM livery, similar to the crash aircraft.AccidentDateJune 19, 1947 (1947-06-19)SummaryEngine fireSiteSyrian Desert, 4 miles (6.4 km) from Mayadin, SyriaAircraftAircraft typeLockheed L-049 ConstellationAircraft nameClipper EclipseOperatorPan American World AirwaysRegistrationNC88845Flight originKarachi Civil Airport, Karachi, British RajDestinationIstanbul, TurkeyOccupants36Passengers26Crew10Fa...

Sceaux 行政国 フランス地域圏 (Région) イル=ド=フランス地域圏県 (département) オー=ド=セーヌ県郡 (arrondissement) アントニー郡小郡 (canton) 小郡庁所在地INSEEコード 92071郵便番号 92330市長(任期) フィリップ・ローラン(2008年-2014年)自治体間連合 (fr) メトロポール・デュ・グラン・パリ人口動態人口 19,679人(2007年)人口密度 5466人/km2住民の呼称 Scéens地理座標 北緯48度4...

Міністерство оборони України (Міноборони) Емблема Міністерства оборони та Прапор Міністерства оборони Будівля Міністерства оборони у КиєвіЗагальна інформаціяКраїна УкраїнаДата створення 24 серпня 1991Попередні відомства Міністерство оборони СРСР Народний комісарі...

2020年夏季奥林匹克运动会波兰代表團波兰国旗IOC編碼POLNOC波蘭奧林匹克委員會網站olimpijski.pl(英文)(波兰文)2020年夏季奥林匹克运动会(東京)2021年7月23日至8月8日(受2019冠状病毒病疫情影响推迟,但仍保留原定名称)運動員206參賽項目24个大项旗手开幕式:帕维尔·科热尼奥夫斯基(游泳)和马娅·沃什乔夫斯卡(自行车)[1]闭幕式:卡罗利娜·纳亚(皮划艇)...
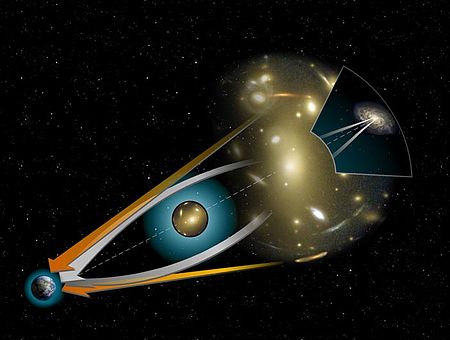
Lentille gravitationnelle pleine. Une étoile à préon est un type d’étoile compacte hypothétique constituée de préons, un groupe de particules subatomiques elles-mêmes hypothétiques. Les étoiles à préon devraient avoir une densité énorme, supérieure à 1023 kg/m3. Il s’agit d’un chiffre intermédiaire entre les étoiles à quarks et les trous noirs. Une étoile à préon d’une masse comparable à celle de la Terre aurait ainsi la taille d’une balle de tennis. Les étoi...
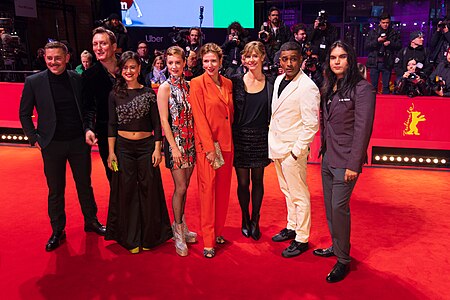
Canadian actor Joshua Odjick and other cast of The Swarm on the red carpet at the Berlinale 2023 (first from the right) Joshua Odjick is a Canadian actor from Kitigan Zibi, Quebec.[1] He is most noted for his performance as Pasmay in the 2021 film Wildhood, for which he won the Canadian Screen Award for Best Supporting Actor at the 10th Canadian Screen Awards in 2022,[2] and the Vancouver Film Critics Circle award for Best Supporting Actor in a Canadian Film at the Vancouver F...

Mass shooting in Norway 2022 Oslo shootingThe police blockade at the location where the shooting took placeLondon PubLondon Pub (Oslo)Show map of OsloLondon PubLondon Pub (Norway)Show map of NorwayLondon PubLondon Pub (Europe)Show map of EuropeLocationOslo, NorwayCoordinates59°54′55″N 10°44′26″E / 59.91528°N 10.74056°E / 59.91528; 10.74056Date25 June 2022 (CEST UTC+02:00)Attack typeMass shootingWeapons MP 40 submachine gun[1] Luger P08 pistol[2&...

Sazeman-e Ettela'at va Amniyat-e KeshvarInformasi lembagaDibentuk1957Dibubarkan1979Lembaga penggantiKantor Intelijen Perdana MenteriKantor pusatTeheranPegawai5000 pada puncaknya[1]MenteriIntelijenPejabat eksekutifTeymur Bakhtiar (Pertama)Nasser Moghadam (Terakhir) SAVAK (Persia: ساواک, singkatan dari سازمان اطلاعات و امنیت کشور Sāzemān-e Ettelā'āt va Amniyat-e Keshvar, secara harfiah Organisasi Keamanan dan Intelijen Nasional) adalah dinas po...
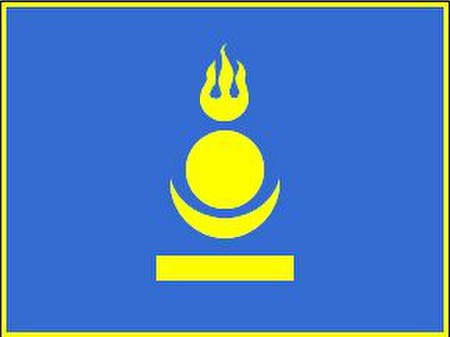
الإمام القاضي الفاضل معلومات شخصية اسم الولادة عبد الرحيم البيساني الميلاد 2 أبريل 1135 عسقلان الوفاة 25 يناير 1200 (64 سنة) القاهرة الحياة العملية المهنة شاعر، وأديب، وكاتب، وسياسي اللغات العربية تعديل مصدري - تعديل عبد الرحيم البيساني، ال�...

Group of baseball parks in the New York City borough of Brooklyn This article is about the baseball stadiums in Brooklyn, New York. For the stadium in Indianapolis, see Washington Park (Indianapolis). For the stadium in Los Angeles, see Washington Park (Los Angeles). Washington ParkEntrance to the second incarnation of Washington Park, 1911Washington ParkLocation in New York CityShow map of New York CityWashington ParkLocation within the State of New YorkShow map of New YorkWashington ParkLoc...

Stefan Bradl pada tahun 2010 Stefan Bradl (lahir 29 November 1989) merupakan seorang pembalap MotoGP berkebangsaan Jerman yang saat ini membalap untuk tim Honda LCR. Dia merupakan juara dunia kelas Moto2 pada tahun 2011. Tahun 2019, Bradl bergabung dengan Repsol Honda sebagai test rider menggaantikan Jorge Lorenzo Biografi Sebelumnya dia mulai membalap di kelas 125cc pada tahun 2005 dan 2006 dia menjadi sebagai seorang wildcard rider untuk tiga seri bersama KTM. Bradl mendapat tawaran dari Al...