Ramer–Douglas–Peucker algorithm
|
Read other articles:

Frederik Willem de Klerk(1990) Nama dalam bahasa asli(af) Frederik Willem de Klerk BiografiKelahiran18 Maret 1936 Johannesburg Kematian11 November 2021 (85 tahun)Kota Tanjung Penyebab kematianMesotelioma 1r Daftar Wakil Presiden Afrika Selatan 10 Mei 1994 – 30 Juni 1996 ← Alwyn Schlebusch (en) – Thabo Mbeki → 7è State President of South Africa (en) 15 Agustus 1989 – 10 Mei 1994 ← Pieter Willem Botha – Nelson M...

US Alessandria Calcio 1912Calcio I Grigi, L'Orso grigio, Mandrogni Segni distintivi Uniformi di gara Casa Trasferta Colori sociali Grigio Simboli Orso Grigio Inno Forza Alessandria Dati societari Città Alessandria Nazione Italia Confederazione UEFA Federazione FIGC Campionato Serie C Fondazione 1912 Rifondazione2003 Proprietario Alessandria 2023 S.r.l. Presidente Andrea Molinaro Allenatore Jonatan Binotto Stadio Giuseppe Moccagatta(5 827 posti) Sito web www.alessandriacalcio1912....

Guerre seminoleVillaggio SeminoleData1817 - 1858 LuogoFlorida, Stati Uniti EsitoEsaurimento, con la maggior parte della tribù deportata in Oklahoma, e una parte minore rimasta libera nelle paludi fino ai giorni nostri[1] Schieramenti Stati UnitiSeminole Yuchi Choctaw Liberti Comandanti Andrew Jackson Martin Van Buren William Henry Harrison John Tyler Duncan Lamont Clinch Edmund P. Gaines Winfield Scott Thomas Jesup Richard Gentry † David Moniac † Francis Langhorne Dade † Zachar...
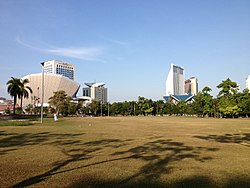
Halaman ini berisi artikel tentang negara bagian di Malaysia. Untuk kegunaan lain, lihat Selangor (disambiguasi). Selangor سلاڠور دار الإحسانNegara bagianKerajaan Negeri Selangor Darul Ehsan[b]Dari atas, kiri ke kanan: Masjid Sultan Salahuddin Abdul Aziz, gemerlap i-City di malam hari, Alun-alun kota Shah Alam, kawasan Batu Caves dengan patung terkenalnya Dewa Murugan, tribun penonton di Sirkuit Internasional Sepang, pematang sawah di Sekinchan BenderaLambang kebesaran...

Voce principale: Savona 1907 Foot-Ball Club. Savona 1907 Foot-Ball ClubStagione 2011-2012Sport calcio Squadra Savona Allenatore Ninni Corda Presidente Aldo Dellepiane Lega Pro Seconda Divisione13º posto 2010-2011 2012-2013 Si invita a seguire il modello di voce Questa voce raccoglie le informazioni riguardanti il Savona 1907 Foot-Ball Club nelle competizioni ufficiali della stagione 2011-2012. Indice 1 Stagione 2 Rosa 3 Risultati 3.1 Campionato 3.1.1 Girone di andata 3.1.2 Girone di ri...

Uomo meditante - Autoritratto del 1467 Nikolaus Gerhaert, detto Nikolaus von Leyden (Leida, 1420 – Vienna, 11 giugno 1473), è stato uno scultore olandese. Indice 1 Biografia 2 Note 3 Bibliografia 4 Altri progetti 5 Collegamenti esterni Biografia Crocifisso nella Collegiata di Baden-Baden, 1467. Lavorò soprattutto in Germania - si hanno informazioni certe dei suoi soggiorni a Treviri, Strasburgo, Costanza e Vienna, tra il 1462 e il 1473. Viene considerato dai critici d'arte come uno degli ...

Leopoldo di Anhalt-KöthenDipinto raffigurante il principe Leopoldo di Anhalt-Köthen col suo kammermohrPrincipe di Anhalt-KöthenStemma In carica30 maggio 1704 –19 novembre 1728 PredecessoreEmmanuele Lebrecht di Anhalt-Köthen SuccessoreAugusto Luigi di Anhalt-Köthen NascitaKöthen, 28 novembre 1694 MorteKöthen, 19 novembre 1728 (33 anni) DinastiaAnhalt-Köthen PadreEmanuele Lebrecht di Anhalt-Köthen MadreGisella Agnese von Rath ConsortiFederica Enrichetta di Anhalt-Bern...
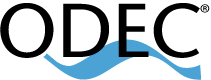
Utility company based in Virginia, United States Old Dominion Electric CooperativeCompany typeCooperative FederationFounded1948 (1948)HeadquartersGlen Allen, Virginia, United StatesArea servedVirginia, Maryland, & DelawareKey peopleMarcus Harris, President & CEORevenue300 MillionMembers11Number of employees104Websiteodec.com Old Dominion Electric Cooperative (ODEC) is an electric generation and transmission cooperative headquartered in Glen Allen, Virginia. ODEC provides wholesal...

جميلة إسماعيل معلومات شخصية اسم الولادة جميلة محمد إسماعيل محمد الميلاد 12 أبريل 1966 (58 سنة) القاهرة مواطنة مصر الحياة العملية المدرسة الأم جامعة القاهرة المهنة صحافية، وسياسية، وناشطة حقوق الإنسان، وكاتِبة الحزب حزب الدستور اللغة الأم الع...
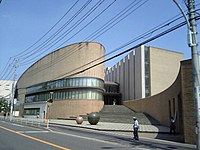
Private educational institution in Kanagawa, Japan This article needs additional citations for verification. Please help improve this article by adding citations to reliable sources. Unsourced material may be challenged and removed.Find sources: Senzoku Gakuen – news · newspapers · books · scholar · JSTOR (November 2013) (Learn how and when to remove this message) Senzoku Gakuen College of Musicand Senzoku Gakuen Junior College洗足学園音楽大学 ...

Tour de Catalogne 1939GénéralitésCourse 19e Tour de CatalogneÉtapes 7 étapesDate 17 au 24 septembre 1939Distance 891 kmPays traversé(s) EspagneLieu de départ BarceloneLieu d'arrivée BarceloneRésultatsVainqueur Mariano Cañardo (ESP)Deuxième Diego CháferTroisième Fermín TruebaMeilleur grimpeur Federico Ezquerra (ESP)Tour de Catalogne 1936Tour de Catalogne 1940modifier - modifier le code - modifier Wikidata Le Tour de Catalogne 1939 est la 19e édition du Tour de Catalogne, un...
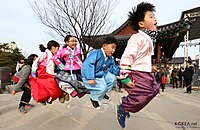
هذه المقالة بحاجة لصندوق معلومات. فضلًا ساعد في تحسين هذه المقالة بإضافة صندوق معلومات مخصص إليها.Learn how and when to remove this message يعتمد التقويم الكوري على القمر أي أنه تقويم قمري، وتم استبدال التقويم الكوري بالتقويم الميلادي عام 1895، لكن لايزال استخدام التقويم الكوري في الاحتفالا�...

هذه المقالة بحاجة لصندوق معلومات. فضلًا ساعد في تحسين هذه المقالة بإضافة صندوق معلومات مخصص إليها. ملصق دستور كوبا كانت كوبا تمتلك عدة دساتير حتى قبل حصولها على استقلالها عن إسبانيا، سواء كانت دساتير اعتمدها الثوار أو اقترحوها كوثائق حاكمة للأراضي التي سيطروا عليها خلال ح...

Part of a series on theOlympic water polorecords and statistics Topics Overall statistics men women Champions men women Team appearances men women Player appearances men women Medalists men women Top goalscorers men women Goalkeepers men women Flag bearers and oath takers Venues Teams Men's teams Australia Belgium Brazil Canada Croatia Egypt France Germany Great Britain Greece Hungary Italy Japan Kazakhstan Montenegro Netherlands Romania Russia Serbia Serbia and Montenegro Soviet Union Spain...

Piers MorganMorgan di acara PaleyFest 2013LahirPiers Stefan O'Meara30 Maret 1965 (umur 59)Newick, Sussex, Inggris[1]KebangsaanBritaniaPendidikanSekolah ChaileyAlmamaterHarlow CollegePekerjaanPresenter televisi, penulis, jurnalisTahun aktif1985–sekarangTempat kerjaSouth London News (1985–88)The Sun (1989–94)News of the World (1994–95)Daily Mirror (1995–2004)Dikenal atasPenyunting koranTuan rumah acara televisiTelevisiBritain's Got TalentAmerica's Got TalentThe Cele...

This article is about the automobile engine. For the steam locomotive, see GWR Iron Duke Class. This article needs additional citations for verification. Please help improve this article by adding citations to reliable sources. Unsourced material may be challenged and removed.Find sources: Iron Duke engine – news · newspapers · books · scholar · JSTOR (January 2014) (Learn how and when to remove this message) Reciprocating internal combustion engine Ir...
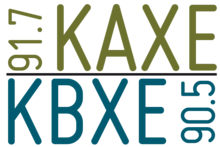
Radio station in Grand Rapids, MinnesotaKAXEGrand Rapids, MinnesotaFrequency91.7 MHzBrandingNorthern Community RadioProgrammingFormatCommunity/PublicAffiliationsNPR, AMPERSOwnershipOwnerNorthern Community RadioHistoryFirst air dateApril 23, 1976[1]Call sign meaningKAXE The Cutting EdgeTechnical informationClassC1ERP100,000 wattsHAAT140 meters (460 ft)Translator(s)see belowRepeater(s)90.5 KBXE (Bagley)LinksWebcastListen LiveWebsitekaxe.org KAXE (91.7 FM) is a community licensed pu...

Hoạt hình của quả bóng nảy lên xuống (dưới đây) bao gồm 6 hình. Hoạt hình này được nhắc lại 10 hình trong một giây. Hoạt hình này chuyển động với tốc độ 2 hình trong một giây. Với tốc độ này, chúng ta hầu như có thể phân biệt được từng hình mộtTốc độ 12 hình trong một giây là tốc độ mà các phim hoạt họa dùng hoạt hình (animated cartoon) thực hiện. Phim hoạt hình hay phim hoạt...

Портал:Политика Россия Статья из серии Политическая системаРоссии Конституция Российской Федерации Всенародное голосование о принятии Конституции (1993) Внесение поправок: • 2008 • февраль 2014 • июль 2014 • 2020 (общероссийское голосование) Основы конституционного строя Нар�...

NeXTstation(コンピュータ) NeXTstation(ネクストステーション)は、1990年から1993年にかけてNeXTが開発・製造・販売した、ワークステーションである。NeXTstationではNEXTSTEPオペレーティングシステムが動作した。NeXTstationは、より手ごろなNeXTcubeとしてリリースされ、価格はおよそ半額のUS $4,995であった。複数のモデル、つまり NeXTstation (25 MHz), NeXTstation Turbo (33 MHz), NeXTstation Color...