Local variable
|
Read other articles:
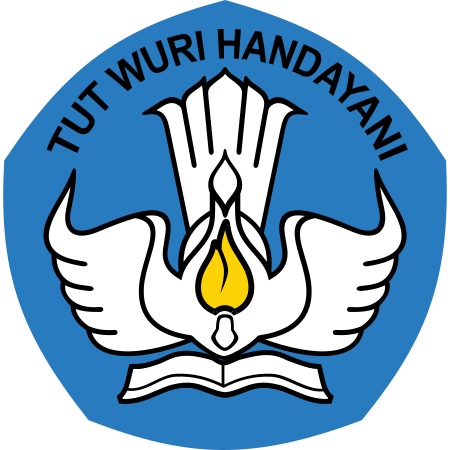
Sekolah Tinggi Ilmu Kesehatan NasionalNama lainSTIKes NasionalJenisPerguruan Tinggi SwastaDidirikan10 Maret 2016KetuaHartono, S.Si., M.Si., Apt.AlamatJl. Raya Solo-Baki, Kwarasan, Grogol, Kabupaten Sukoharjo, Jawa Tengah, 57552, IndonesiaBahasaBahasa IndonesiaSitus webstikesnas.ac.id Sekolah Tinggi Ilmu Kesehatan Nasional (disingkat STIKes Nasional) adalah salah satu perguruan tinggi swasta di Indonesia yang berlokasi di Kabupaten Sukoharjo, Jawa Tengah. Perguruan tinggi ini dikelola oleh Yay...

Prof. Dr.Edy Suandi Hamid M.Ec. Rektor Universitas Widya MataramPetahanaMulai menjabat 4 September 2017 PendahuluProf. Dr. Muchsan, S.H.PenggantiPetahanaRektor Universitas Islam IndonesiaMasa jabatan2006–2014 PendahuluDr. Ir. Luthfi Hasan, MS.PenggantiDr. Ir. Harsoyo, MS. Informasi pribadiLahir11 Desember 1957 (umur 66)Tanjung EnimKebangsaanIndonesiaSuami/istriEmy Rohayati, S.H.Anak Dian Vita Alpha Suandi Aprilia Beta Suandi Paramitha Gama Suandi Ulfa Ramadhani Delta Suandi Muhamma...
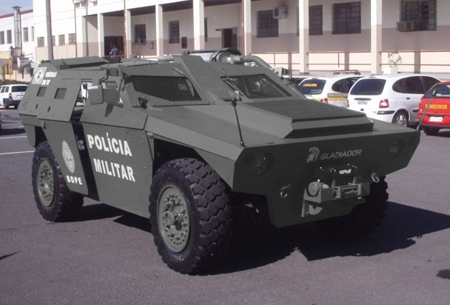
This article includes a list of references, related reading, or external links, but its sources remain unclear because it lacks inline citations. Please help to improve this article by introducing more precise citations. (January 2013) (Learn how and when to remove this template message) Wheeled all-terrain vehicle VBL Gladiador TypeWheeled all-terrain vehiclePlace of origin BrazilSpecificationsMass3.5tLength3.80 m (4.00 m long version)Width2.02 mHeight1.70 mCrew2-3ArmourSTANAG...

Sultan Abul Mafakhir Muhammad Aliuddin II [[Sultan Banten]] 16Masa jabatan1803 – 1808 PendahuluSultan Abul Nashar Muhammad Ishaq ZainulmutaqinPenggantiSultan Maulana Muhammad Shafiuddin Informasi pribadiLahirAbul Mufakhir Muhammad AqiluddinSurosowan, Kesultanan BantenMeninggalAmbon, Hindia BelandaKebangsaanBantenHubunganSultan Abul Mafakhir Muhammad Aliuddin I (Ayah)Sultan Abul Nashar Muhammad Ishaq Zainulmutaqin (Kakak)Pangeran Ahmad al-Qudsi (Adik)Pangeran Dharma (Adik)Panger...

Peta yang menunjukkan distribusi dari keluarga bahasa; warna merah muda yang menunjukkan di mana bahasa Austronesia yang dituturkan. Ini adalah daftar bahasa-bahasa resmi dan utama dari rumpun Austronesia, sebuah rumpun bahasa yang tersebar di seluruh pulau-pulau Asia Tenggara dan Pasifik, ditambah sebagian kecil anggota di Asia daratan dan Madagaskar. Bahasa resmi Negara berdaulat Bahasa Jumlah penutur Nama asli Status resmi Fiji 639,210 Na Vosa Vakaviti Fiji Filipino (Tagalog) 100,000...

Voce principale: Bologna Football Club 1909. Bologna FCStagione 1979-1980 Sport calcio Squadra Bologna Allenatore Marino Perani Presidente Tommaso Fabbretti Serie A7º Coppa ItaliaPrimo turno Maggiori presenzeCampionato: Sali (30) Miglior marcatoreCampionato: Savoldi (11) StadioComunale 1978-1979 1980-1981 Si invita a seguire il modello di voce Questa voce raccoglie le informazioni riguardanti il Bologna Football Club nelle competizioni ufficiali della stagione 1979-1980. Indice 1 Stagi...

Presiden Lula berpidato di hadapan penerima Bolsa Família di Diadema. Bolsa Família (pengucapan bahasa Portugis: [ˈbowsɐ faˈmiliɐ], Tunjangan Keluarga) adalah program kesejahteraan sosial yang dilancarkan oleh pemerintah Brasil. Program ini merupakan bagian dari program bantuan federal Fome Zero. Bolsa Família memberikan bantuan keuangan untuk keluarga Brasil yang miskin; bila mereka punya anak, mereka harus memastikan bahwa anak mereka masuk sekolah dan divaksinasi. Program ini b...

Shahrbaraz𐭧𐭱𐭨𐭥𐭥𐭥𐭰Shah ĒrānshahrBerkuasa27 April 629 – 17 Juni 629PendahuluArdashir IIIPenerusBorandukhtKematian17 Juni 629CtesiphonWangsaWangsa MihranAgamaZoroastrianisme Shahrbaraz, juga disebut Shahrvaraz (Persia: شهربراز, bahasa Persia Pertengahan: 𐭧𐭱𐭨𐭥𐭥𐭥𐭰 Šahrwarāz, meninggal 17 Juni 629), adalah raja Kekaisaran Sassania dari 27 April 629 hingga 17 Juni 629. Ia merebut takhta Ardashir III, tetapi empat puluh hari kemudian dibu...
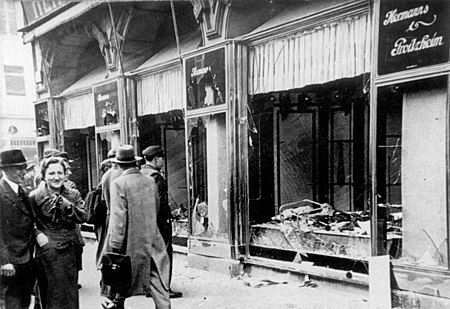
German economist, Nazi politician and convicted war criminal (1890–1960) This article needs additional citations for verification. Please help improve this article by adding citations to reliable sources. Unsourced material may be challenged and removed.Find sources: Walther Funk – news · newspapers · books · scholar · JSTOR (April 2012) (Learn how and when to remove this message) You can help expand this article with text translated from the corresp...

English actor Ellis HollinsHollins in 2019Born (1999-11-14) 14 November 1999 (age 24)Oldham, EnglandOccupationActorYears active1999–presentEmployerLime PicturesTelevisionHollyoaks Ellis Hollins (born 14 November 1999) is an English actor. He is known for portraying the role of Tom Cunningham in the Channel 4 soap opera Hollyoaks. In 2006, he appeared in Alpha Male, a family film released in the United Kingdom. Acting work At age 3, Hollins was cast as Tom Cunningham in Channel 4's...

Super Show 2Tur {{{type}}} oleh Super JuniorPoster promosi untuk Super Show 2Sorry, SorryMulai17 Juli 2009 (2009-07-17)Berakhir10 April 2010 (2010-04-10)Putaran7Penampilan3 di Korea Selatan1 di Hong Kong5 di Tiongkok2 di Thailand2 di Taiwan1 di Malaysia1 di Filipinatotal 15Situs websuperjunior.smtown.comKronologi konser Super Junior Super Show (2008–09) Super Show 2 (2009–10) Super Show 3 (2010–11) Super Show 2 tur konser Asia kedua yang dis...

The following is a list of the 259 communes of the French department of Yvelines. The communes cooperate in the following intercommunalities (as of 2020):[1] CU Grand Paris Seine et Oise CA Cergy-Pontoise (partly) Communauté d'agglomération Rambouillet Territoires Communauté d'agglomération Saint Germain Boucles de Seine (partly) CA Saint-Quentin-en-Yvelines Communauté d'agglomération Versailles Grand Parc (partly) Communauté de communes Cœur d'Yvelines [fr] Comm...

NGC 3100 الكوكبة مفرغة الهواء[1] رمز الفهرس NGC 3100 (الفهرس العام الجديد)PGC 28960 (فهرس المجرات الرئيسية)2MASX J10004083-3139519 (Two Micron All-Sky Survey, Extended source catalogue)MCG-05-24-018 (فهرس المجرات الموروفولوجي)ESO 435-30 (European Southern Observatory Catalog)NGC 3103 (الفهرس العام الجديد)AM 0958-312 (A catalogue of southern peculiar galaxies and associa...

United States national observatoryObservatoryNational Optical Astronomy ObservatoryAlternative namesNOAO OrganizationNational Science Foundation, AURALocationTucson, Pima County, ArizonaEstablished1984Closed2019Websitehttps://legacy.noirlab.edu/about-noao.php Related media on Commons[edit on Wikidata]Kitt Peak is the National Observatory of the United States, in contrast to the various benefactor and privately funded telescopes. The largest optical telescope at Kitt Peak i...

Sommerkahl Lambang kebesaranLetak Sommerkahl di Aschaffenburg NegaraJermanNegara bagianBayernWilayahUnterfrankenKreisAschaffenburgMunicipal assoc.Schöllkrippen Subdivisions2 OrtsteilePemerintahan • MayorArnold Markert (CSU)Luas • Total5,46 km2 (211 sq mi)Ketinggian226 m (741 ft)Populasi (2013-12-31)[1] • Total1.131 • Kepadatan2,1/km2 (5,4/sq mi)Zona waktuWET/WMPET (UTC+1/+2)Kode pos63825Kode area telep...

Questa voce sull'argomento centri abitati del Rio Grande do Sul è solo un abbozzo. Contribuisci a migliorarla secondo le convenzioni di Wikipedia. Segui i suggerimenti del progetto di riferimento. Iraícomune Iraí – Veduta LocalizzazioneStato Brasile Stato federato Rio Grande do Sul MesoregioneNoroeste Rio-Grandense MicroregioneFrederico Westphalen AmministrazioneSindacoVolmir Bielski TerritorioCoordinate27°11′40″S 53°15′18″W27°11′40″S, 53°15′18″W (Ir...

Main article: 1996 United States presidential election 1996 United States presidential election in Montana ← 1992 November 5, 1996 (1996-11-05) 2000 → Nominee Bob Dole Bill Clinton Ross Perot Party Republican Democratic Reform Home state Kansas Arkansas Texas Running mate Jack Kemp Al Gore James Campbell Electoral vote 3 0 0 Popular vote 179,652 167,922 55,229 Percentage 44.11% 41.23% 13.56% County Results Dole 40-50...

Blå ängeln(Der blaue Engel)GenreDrama, MusikalRegissörJosef von SternbergProducentErich PommerManusHeinrich MannCarl ZuckmayerKarl VollmöllerRobert LiebmannSkådespelareEmil JanningsMarlene DietrichHans AlbersKurt GerronRosa ValettiEduard von WintersteinOriginalmusikFriedrich Hollaender,Wolfgang Amadeus MozartPremiär1 april 1930 (Tyskland) 17 november 1930 (Sverige)Speltid99 minuterLandTysklandSpråkTyskaIMDb SFDb Elonet För nyinspelningen från 1959, se Blå ängeln (film, 1959) Blå �...

Australien Bureau of Meteorology— BOM — Stellung der Behörde Nationaler meteorologischer Dienst Aufsichtsbehörde(n) Ministry for Environment, Heritage and the Arts Bestehen seit 1906 Hauptsitz Melbourne Behördenleitung Andrew Johnson (Director of Meteorology)[1][2] Website www.bom.gov.au Das Bureau of Meteorology ist ein Exekutivorgan der Bundesregierung Australiens und verantwortlich für die Erstellung von Wettervorhersagen für Australien und di...

世論では第二次世界大戦後から日本は追従するようになったと思われがちだが、明治時代や大正時代から既にアメリカ合衆国及び大英帝国に追従する国だったと言われていた(これはハワイ併合時(1897年)の風刺画である)。アメリカ合衆国(アンクル・サム)が 「なぜこの奇妙な猟犬(大日本帝国)はどこに行くにも私(アメリカ合衆国)の後を追うの?」、イギリ...