Bitwise operations in C
The bitwise AND operator is a single ampersand: For instance, working with a byte (the char type): 11001000 & 10111000 -------- = 10001000 The most significant bit of the first number is 1 and that of the second number is also 1 so the most significant bit of the result is 1; in the second most significant bit, the bit of second number is zero, so we have the result as 0. [2] Bitwise OR |
bit a | bit b | a | b (a OR b) |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 1 |
Similar to bitwise AND, bitwise OR performs logical disjunction at the bit level. Its result is a 1 if either of the bits is 1 and zero only when both bits are 0. Its symbol is |
which can be called a pipe.
11001000
| 10111000
--------
= 11111000
Bitwise XOR ^
bit a | bit b | a ^ b (a XOR b)
|
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
The bitwise XOR (exclusive or) performs an exclusive disjunction, which is equivalent to adding two bits and discarding the carry. The result is zero only when we have two zeroes or two ones.[3] XOR can be used to toggle the bits between 1 and 0. Thus i = i ^ 1
when used in a loop toggles its values between 1 and 0.[4]
11001000
^ 10111000
--------
= 01110000
Shift operators
There are two bitwise shift operators. They are
- Right shift (
>>
) - Left shift (
<<
)
Right shift >>
The symbol of right shift operator is >>
. For its operation, it requires two operands. It shifts each bit in its left operand to the right.
The number following the operator decides the number of places the bits are shifted (i.e. the right operand).
Thus by doing ch >> 3
all the bits will be shifted to the right by three places and so on.
However, do note that a shift operand value which is either a negative number or is greater than or equal to the total number of bits in this value results in undefined behavior. For example, when shifting a 32 bit unsigned integer, a shift amount of 32 or higher would be undefined.
Example:
- If the variable
ch
contains the bit pattern11100101
, thench >> 1
will produce the result01110010
, andch >> 2
will produce00111001
.
Here blank spaces are generated simultaneously on the left when the bits are shifted to the right. When performed on an unsigned type or a non-negative value in a signed type, the operation performed is a logical shift, causing the blanks to be filled by 0
s (zeros). When performed on a negative value in a signed type, the result is technically implementation-defined (compiler dependent),[5] however most compilers will perform an arithmetic shift, causing the blank to be filled with the set sign bit of the left operand.
Right shift can be used to divide a bit pattern by 2 as shown:
i = 14; // Bit pattern 00001110
j = i >> 1; // here we have the bit pattern shifted by 1 thus we get 00000111 = 7 which is 14/2
Right shift operator usage
Typical usage of a right shift operator in C can be seen from the following code.
Example:
#include <stdio.h>
void showbits( unsigned int x )
{
int i=0;
for (i = (sizeof(int) * 8) - 1; i >= 0; i--)
{
putchar(x & (1u << i) ? '1' : '0');
}
printf("\n");
}
int main( void )
{
int j = 5225;
printf("%d in binary \t\t ", j);
showbits(j);
/* the loop for right shift operation */
for (int m = 0; m <= 5; m++)
{
int n = j >> m;
printf("%d right shift %d gives ", j, m);
showbits(n);
}
return 0;
}
The output of the above program will be
5225 in binary 00000000000000000001010001101001
5225 right shift 0 gives 00000000000000000001010001101001
5225 right shift 1 gives 00000000000000000000101000110100
5225 right shift 2 gives 00000000000000000000010100011010
5225 right shift 3 gives 00000000000000000000001010001101
5225 right shift 4 gives 00000000000000000000000101000110
5225 right shift 5 gives 00000000000000000000000010100011
Left shift <<
The symbol of left shift operator is <<
. It shifts each bit in its left-hand operand to the left by the number of positions indicated by the right-hand operand. It works opposite to that of right shift operator. Thus by doing ch << 1
in the above example (11100101
) we have 11001010
.
Blank spaces generated are filled up by zeroes as above.
However, do note that a shift operand value which is either a negative number or is greater than or equal to the total number of bits in this value results in undefined behavior. This is defined in the standard at ISO 9899:2011 6.5.7 Bit-wise shift operators. For example, when shifting a 32 bit unsigned integer, a shift amount of 32 or higher would be undefined.
Left shift can be used to multiply an integer by powers of 2 as in
int i = 7; // Decimal 7 is Binary (2^2) + (2^1) + (2^0) = 0000 0111
int j = 3; // Decimal 3 is Binary (2^1) + (2^0) = 0000 0011
k = (i << j); // Left shift operation multiplies the value by 2 to the power of j in decimal
// Equivalent to adding j zeros to the binary representation of i
// 56 = 7 * 2^3
// 0011 1000 = 0000 0111 << 0000 0011
Example: a simple addition program
The following program adds two operands using AND, XOR and left shift (<<).
#include <stdio.h>
int main( void )
{
unsigned int x = 3, y = 1, sum, carry;
sum = x ^ y; // x XOR y
carry = x & y; // x AND y
while (carry != 0)
{
carry = carry << 1; // left shift the carry
x = sum; // initialize x as sum
y = carry; // initialize y as carry
sum = x ^ y; // sum is calculated
carry = x & y; /* carry is calculated, the loop condition is
evaluated and the process is repeated until
carry is equal to 0.
*/
}
printf("%u\n", sum); // the program will print 4
return 0;
}
Bitwise assignment operators
C provides a compound assignment operator for each binary arithmetic and bitwise operation. Each operator accepts a left operand and a right operand, performs the appropriate binary operation on both and stores the result in the left operand.[6]
The bitwise assignment operators are as follows.
Symbol | Operator |
---|---|
&= |
bitwise AND assignment |
|= |
bitwise inclusive OR assignment |
^= |
bitwise exclusive OR assignment |
<<= |
left shift assignment |
>>= |
right shift assignment |
Logical equivalents
Four of the bitwise operators have equivalent logical operators. They are equivalent in that they have the same truth tables. However, logical operators treat each operand as having only one value, either true or false, rather than treating each bit of an operand as an independent value. Logical operators consider zero false and any nonzero value true. Another difference is that logical operators perform short-circuit evaluation.
The table below matches equivalent operators and shows a and b as operands of the operators.
Bitwise | Logical |
---|---|
a & b |
a && b
|
a | b |
a || b
|
a ^ b |
a != b
|
~a |
!a
|
!=
has the same truth table as ^
but unlike the true logical operators, by itself !=
is not strictly speaking a logical operator. This is because a logical operator must treat any nonzero value the same. To be used as a logical operator !=
requires that operands be normalized first. A logical not applied to both operands will not change the truth table that results but will ensure all nonzero values are converted to the same value before comparison. This works because !
on a zero always results in a one and !
on any nonzero value always results in a zero.
Example:
/* Equivalent bitwise and logical operator tests */
#include <stdio.h>
void testOperator(char* name, unsigned char was, unsigned char expected);
int main( void )
{
// -- Bitwise operators -- //
//Truth tables packed in bits
const unsigned char operand1 = 0x0A; //0000 1010
const unsigned char operand2 = 0x0C; //0000 1100
const unsigned char expectedAnd = 0x08; //0000 1000
const unsigned char expectedOr = 0x0E; //0000 1110
const unsigned char expectedXor = 0x06; //0000 0110
const unsigned char operand3 = 0x01; //0000 0001
const unsigned char expectedNot = 0xFE; //1111 1110
testOperator("Bitwise AND", operand1 & operand2, expectedAnd);
testOperator("Bitwise OR", operand1 | operand2, expectedOr);
testOperator("Bitwise XOR", operand1 ^ operand2, expectedXor);
testOperator("Bitwise NOT", ~operand3, expectedNot);
printf("\n");
// -- Logical operators -- //
const unsigned char F = 0x00; //Zero
const unsigned char T = 0x01; //Any nonzero value
// Truth tables packed in arrays
const unsigned char operandArray1[4] = {T, F, T, F};
const unsigned char operandArray2[4] = {T, T, F, F};
const unsigned char expectedArrayAnd[4] = {T, F, F, F};
const unsigned char expectedArrayOr[4] = {T, T, T, F};
const unsigned char expectedArrayXor[4] = {F, T, T, F};
const unsigned char operandArray3[2] = {F, T};
const unsigned char expectedArrayNot[2] = {T, F};
int i;
for (i = 0; i < 4; i++)
{
testOperator("Logical AND", operandArray1[i] && operandArray2[i], expectedArrayAnd[i]);
}
printf("\n");
for (i = 0; i < 4; i++)
{
testOperator("Logical OR", operandArray1[i] || operandArray2[i], expectedArrayOr[i]);
}
printf("\n");
for (i = 0; i < 4; i++)
{
//Needs ! on operand's in case nonzero values are different
testOperator("Logical XOR", !operandArray1[i] != !operandArray2[i], expectedArrayXor[i]);
}
printf("\n");
for (i = 0; i < 2; i++)
{
testOperator("Logical NOT", !operandArray3[i], expectedArrayNot[i]);
}
printf("\n");
return 0;
}
void testOperator( char* name, unsigned char was, unsigned char expected )
{
char* result = (was == expected) ? "passed" : "failed";
printf("%s %s, was: %X expected: %X \n", name, result, was, expected);
}
The output of the above program will be
Bitwise AND passed, was: 8 expected: 8
Bitwise OR passed, was: E expected: E
Bitwise XOR passed, was: 6 expected: 6
Bitwise NOT passed, was: FE expected: FE
Logical AND passed, was: 1 expected: 1
Logical AND passed, was: 0 expected: 0
Logical AND passed, was: 0 expected: 0
Logical AND passed, was: 0 expected: 0
Logical OR passed, was: 1 expected: 1
Logical OR passed, was: 1 expected: 1
Logical OR passed, was: 1 expected: 1
Logical OR passed, was: 0 expected: 0
Logical XOR passed, was: 0 expected: 0
Logical XOR passed, was: 1 expected: 1
Logical XOR passed, was: 1 expected: 1
Logical XOR passed, was: 0 expected: 0
Logical NOT passed, was: 1 expected: 1
Logical NOT passed, was: 0 expected: 0
See also
- Bit manipulation
- Bitwise operation
- Find first set
- Operators in C and C++
- Bitboard
- Boolean algebra (logic)
- XOR swap algorithm
- XOR linked list
References
- ^ Kernighan; Dennis M. Ritchie (March 1988). The C Programming Language (2nd ed.). Englewood Cliffs, NJ: Prentice Hall. ISBN 0-13-110362-8. Archived from the original on 2019-07-06. Retrieved 2019-09-07. Regarded by many to be the authoritative reference on C.
- ^ a b "Tutorials - Bitwise Operators and Bit Manipulations in C and C++". cprogramming.com.
- ^ "Exclusive-OR Gate Tutorial". Basic Electronics Tutorials.
- ^ "C++ Notes: Bitwise Operators". fredosaurus.com.
- ^ "ISO/IEC 9899:2011 - Information technology -- Programming languages -- C". www.iso.org.
- ^ "Compound assignment operators". IBM. International Business Machines. Retrieved 29 January 2022.
External links
Read other articles:

Struktur trigliserilda dengan gliserol sebagai rantai utama Minyak adalah istilah umum untuk semua cairan organik yang tidak larut/bercampur dalam air (hidrofobik) tetapi larut dalam pelarut organik.[1] Ada sifat tambahan lain yang dikenal awam: terasa licin apabila dipegang.[1] Dalam arti sempit, kata 'minyak' biasanya mengacu ke minyak bumi (petroleum) atau produk olahannya: minyak tanah (kerosena).[1] Namun, kata ini sebenarnya berlaku luas, baik untuk minyak sebaga...

Часть серии статей о Холокосте Идеология и политика Расовая гигиена · Расовый антисемитизм · Нацистская расовая политика · Нюрнбергские расовые законы Шоа Лагеря смерти Белжец · Дахау · Майданек · Малый Тростенец · Маутхаузен ·&...

TōjinbōIUCN category IV (habitat/species management area)Sandan Rocks and Byobu RocksLocation of TōjinbōShow map of Fukui PrefectureTōjinbō (Japan)Show map of JapanLocationSakai, Fukui, JapanCoordinates36°14′17″N 136°07′30″E / 36.238°N 136.125°E / 36.238; 136.125Established1935 Tōjinbō (東尋坊) is a series of cliffs on the Sea of Japan in Japan. It is located in the Antō part of Mikuni-chō in Sakai, Fukui Prefecture. The cliffs average 30 metre...
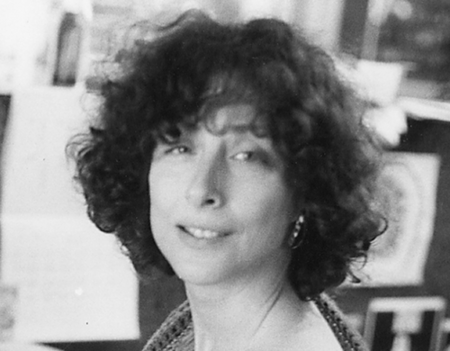
Collective debates amongst feminists regarding a number of issues The examples and perspective in this article may not represent a worldwide view of the subject. You may improve this article, discuss the issue on the talk page, or create a new article, as appropriate. (December 2017) (Learn how and when to remove this template message) Part of a series onFeminism History Feminist history History of feminism Women's history American British Canadian German Waves First Second Third Fourth Timel...

Синелобый амазон Научная классификация Домен:ЭукариотыЦарство:ЖивотныеПодцарство:ЭуметазоиБез ранга:Двусторонне-симметричныеБез ранга:ВторичноротыеТип:ХордовыеПодтип:ПозвоночныеИнфратип:ЧелюстноротыеНадкласс:ЧетвероногиеКлада:АмниотыКлада:ЗавропсидыКласс:Пт�...

Questa voce o sezione sull'argomento geologia non cita le fonti necessarie o quelle presenti sono insufficienti. Puoi migliorare questa voce aggiungendo citazioni da fonti attendibili secondo le linee guida sull'uso delle fonti. Segui i suggerimenti del progetto di riferimento. Spessore della crosta terrestre (in km) La crosta terrestre (chiamata comunemente superficie terrestre), in geologia e in geofisica, è uno degli involucri concentrici di cui è costituita la Terra: per la precis...

County in Kentucky, United States County in KentuckyBullitt CountyCountyBullitt County Courthouse in Shepherdsville FlagSealLocation within the U.S. state of KentuckyKentucky's location within the U.S.Coordinates: 37°58′N 85°42′W / 37.97°N 85.7°W / 37.97; -85.7Country United StatesState KentuckyFounded1792Named forAlexander Scott BullittSeatShepherdsvilleLargest cityMount WashingtonArea • Total300 sq mi (800 km2) •...

This article has multiple issues. Please help improve it or discuss these issues on the talk page. (Learn how and when to remove these template messages) This article needs additional citations for verification. Please help improve this article by adding citations to reliable sources. Unsourced material may be challenged and removed.Find sources: List of Tour de France secondary classification winners – news · newspapers · books · scholar · JSTOR (May...

Government debt security issued to finance wartime expenditure United Kingdom national war bond advertisement (1918) War bonds (sometimes referred to as victory bonds, particularly in propaganda) are debt securities issued by a government to finance military operations and other expenditure in times of war without raising taxes to an unpopular level. They are also a means to control inflation by removing money from circulation in a stimulated wartime economy.[1] War bonds are either r...

この項目には、一部のコンピュータや閲覧ソフトで表示できない文字が含まれています(詳細)。 数字の大字(だいじ)は、漢数字の一種。通常用いる単純な字形の漢数字(小字)の代わりに同じ音の別の漢字を用いるものである。 概要 壱万円日本銀行券(「壱」が大字) 弐千円日本銀行券(「弐」が大字) 漢数字には「一」「二」「三」と続く小字と、「壱」「�...

Флаг гордости бисексуалов Бисексуальность Сексуальные ориентации Бисексуальность Пансексуальность Полисексуальность Моносексуальность Сексуальные идентичности Би-любопытство Гетерогибкость и гомогибкость Сексуальная текучесть Исследования Шк...

Administrative entry restrictions An Armenian passport Visa requirements for Armenian citizens are administrative entry restrictions by the authorities of other states placed on citizens of Armenia. As of January 2024, Armenian citizens had visa-free or visa on arrival access to 69 countries and territories, ranking the Armenian passport 75th in terms of travel freedom according to the Henley Passport Index.[1] Visa requirements map Visa requirements for Armenian citizens holding ordi...

Tong MarimbunKelurahanKantor Kelurahan Tong MarimbunPeta lokasi Kelurahan Tong MarimbunNegara IndonesiaProvinsiSumatera UtaraKotaPematangsiantarKecamatanSiantar MarimbunKode Kemendagri12.72.08.1002 Kode BPS1273011004 Luaskm²Kepadatan- Tong Marimbun adalah salah satu kelurahan di Kecamatan Siantar Marimbun, Pematangsiantar, Sumatera Utara, Indonesia. Galeri Gapura selamat datang di Kelurahan Tong Marimbun Gereja GKPI Siantar Sawah di Kelurahan Tong Marimbun Gereja HKBP Siantar Sawah di K...

Religion in Uganda (2014 census)[1][2] Catholicism (39.3%) Anglicanism (32.0%) Pentecostalism (11.1%) Other Christian (2.0%) Islam (13.7%) No religion (1.2%) Others (0.7%) St. Paul's Anglican Cathedral in the capital Kampala Christianity is the predominant religion in Uganda. According to the 2014 census, over 84 percent of the population was Christian, while about 14 percent of the population adher...
Oasis region in Central Asia Not to be confused with Khorasan. Khorezm redirects here. For the Soviet republic, see Khorezm People's Soviet Republic. For the coextensive state which preceded it, see Khanate of Khiva. For the modern-day region of Uzbekistan, see Xorazm Region. For other uses, see Khwarezmian (disambiguation). Khwarazm(Chorasmia)KhwarazmLocation of the Khwarazm heartland in Central AsiaMap of Khwarazm during the early Islamic periodToday part ofUzbekistanTurkmenistan Khwarazm (...

Film production company This article needs additional citations for verification. Please help improve this article by adding citations to reliable sources. Unsourced material may be challenged and removed.Find sources: BBC Film – news · newspapers · books · scholar · JSTOR (August 2023) (Learn how and when to remove this message) BBC FilmFormerlyBBC Films (1990–2020)IndustryFilmFounded18 June 1990; 33 years ago (18 June 1990)FoundersDavi...

Summer in ParisSingel oleh DJ Cam featuring Anggundari album SoulshineDirilis2002FormatCD SingleDirekam2002GenreJazz, ClubLabelSony MusicProduserDJ Cam Summer in Paris adalah singel dari Anggun yang berkolaborasi bersama DJ Cam pada tahun 2002. Di dalam lagu jazzy ini Anggun menjadi vokalis tamu yang diiringi oleh hentakan musik DJ Cam. Lagu ini sendiri resminya dirilis di album DJ Cam bertajuk Soulshine pada tahun 2002. Anggun juga memuat ulang lagu ini di album Best Of-nya pada tahun 2006. ...

Pour les articles homonymes, voir Iwata (homonymie). Cet article est une ébauche concernant une localité japonaise. Vous pouvez partager vos connaissances en l’améliorant (comment ?) selon les recommandations des projets correspondants. Iwata-shi 磐田市 Mairie d'Iwata. Drapeau Administration Pays Japon Région Chūbu Préfecture Shizuoka Maire Osamu Watanabe Code postal 〒438-8650 Démographie Population 169 873 hab. (août 2019) Densité 1 039 hab./km2 Géo...

Medieval map Nuzhat al-mushtāq fī ikhtirāq al-āfāq (نزهة المشتاق في اختراق الآفاق) Tabula Rogeriana Map of al-Maghrib al-Aqsa and al-Maghrib al-Awsat (south-up) in MS arabe 2221, the oldest known surviving manuscript copy of Idrisi's Tabula Rogeriana.AuthorMuhammad al-IdrisiMedia typeAtlas The Nuzhat al-mushtāq fī ikhtirāq al-āfāq (Arabic: نزهة المشتاق في اختراق الآفاق, lit. The Excursion of One Eager to Penetrate the Distant Ho...

Pour les articles homonymes, voir Rattray. Frank Rattray LillieBiographieNaissance 27 juin 1870TorontoDécès 5 novembre 1947 (à 77 ans)ChicagoNationalité américaineFormation Université de TorontoVassar CollegeActivités Zoologiste, scientifique, embryologiste, professeur d'universitéAutres informationsA travaillé pour Université de ChicagoUniversité du MichiganVassar CollegeMembre de Académie américaine des sciencesAcadémie américaine des arts et des sciencesDistinction Mé...