Jakarta Mail
|
Read other articles:

العلاقات الروسية المنغولية منغوليا روسيا السفارات سفارة روسيا في منغوليا السفير : إسكندر أزيزوف[1] سفارة منغوليا في روسيا السفير : شوخيرييْن ألتانغيريل[2] تعديل مصدري - تعديل العلاقات الروسية المنغولية هي العلاقات الثنائية بين ج�...

Artikel ini bukan mengenai Kereta api Madiun Jaya Ekspres. Kereta api Madiun Ekspres singgah di stasiun Mojokerto. KA Madiun Ekspres merupakan KA eksekutif, dan bisnis tujuan Madiun-Surabaya PP. KA Madiun Ekspres berhenti di beberapa stasiun besar, antara lain Nganjuk, Kertosono, Jombang, dan Mojokerto. Stamformasi kereta ini hingga akhir operasionalnya ialah, 2 kereta bisnis (K2), 1 kereta makan pembangkit (KMP), 1 kereta eksekutif (K1), dan lokomotif BB301. Kereta api ini berangkat menuju S...

2 Tawarikh 10Kitab Tawarikh (Kitab 1 & 2 Tawarikh) lengkap pada Kodeks Leningrad, dibuat tahun 1008.KitabKitab 2 TawarikhKategoriKetuvimBagian Alkitab KristenPerjanjian LamaUrutan dalamKitab Kristen14← pasal 9 pasal 11 → 2 Tawarikh 10 (atau II Tawarikh 10, disingkat 2Taw 10) adalah bagian dari Kitab 2 Tawarikh dalam Alkitab Ibrani dan Perjanjian Lama di Alkitab Kristen. Dalam Alkitab Ibrani termasuk dalam bagian Ketuvim (כְּתוּבִים, tulisan).[1][2] Te...

Cet article est une ébauche concernant un physicien chinois. Vous pouvez partager vos connaissances en l’améliorant (comment ?) selon les recommandations des projets correspondants. Bei ShizhangBei Shizhang en 1930FonctionsMembre du comité national de la conférence consultative politique du peuple chinois3e comité national de la conférence consultative politique du peuple chinois (d)Député6e Assemblée nationale populaire (en)5e Assemblée nationale populaire (en)4e Assemblée...

Pleione in the Pleiades is a shell star. A shell star is a star having a spectrum that shows extremely broad absorption lines, plus some very narrow absorption lines. They typically also show some emission lines, usually from the Balmer series but occasionally of other lines. The broad absorption lines are due to rapid rotation of the photosphere, the emission lines from an equatorial disk, and the narrow absorption lines are produced when the disc is seen nearly edge-on. Shell stars have spe...
Geoff Stults nel 2018 Geoffrey Manton Stults (Detroit, 15 dicembre 1977) è un attore ed ex giocatore di football americano statunitense. Indice 1 Biografia 2 Football americano 3 Filmografia parziale 3.1 Cinema 3.2 Televisione 4 Doppiatori italiani 5 Altri progetti 6 Collegamenti esterni Biografia Cresciuto in Colorado, si trasferisce a Los Angeles e comincia a recitare a teatro mentre studia allo Whittier College. Giocatore di football professionista, ha giocato come ricevitore nei Klostern...

هنودمعلومات عامةنسبة التسمية الهند التعداد الكليالتعداد قرابة 1.21 مليار[1][2]تعداد الهند عام 2011ق. 1.32 مليار[3]تقديرات عام 2017ق. 30.8 مليون[4]مناطق الوجود المميزةبلد الأصل الهند البلد الهند الهند نيبال 4,000,000[5] الولايات المتحدة 3,982,398[6] الإمار...

Headland in Sydney, New South Wales, Australia 33°51′9″S 151°14′45″E / 33.85250°S 151.24583°E / -33.85250; 151.24583 Location of the antagonist's Sydney residence in Mission: Impossible 2. The house itself has been removed. Bradleys Head is a headland protruding from the north shore of Sydney Harbour, within the metropolitan area of Sydney, New South Wales, Australia. It is named after the First Fleet naval officer William Bradley. The original Aboriginal i...

本文或本章節是關於未來的公共运输建設或計划。未有可靠来源的臆測內容可能會被移除,現時內容可能與竣工情況有所出入。 此条目讲述中国大陆處於施工或详细规划阶段的工程。设计阶段的資訊,或許与竣工后情況有所出入。无可靠来源供查证的猜测会被移除。 设想中的三条路线方案[1]。 臺灣海峽隧道或臺湾海峡橋隧(英語:Taiwan Strait Tunnel Project)是一项工程�...

Para el circuito permanente, véase Circuito de Shanghái. Circuito Urbano de Shanghái Trazado actualUbicación Shanghái, China 31°13′16″N 121°32′38″E / 31.22111, 121.54389Coordenadas 31°13′16″N 121°32′38″E / 31.22111111, 121.54388889Eventos DTMLongitud 3,1 kmCurvas 9[editar datos en Wikidata] El Circuito Urbano de Shanghái fue un circuito no permanente situado en el distrito de Pudong, cerca del distrito financiero de Shanghá...
الثقافة الأعلام والتراجم الجغرافيا التاريخ الرياضيات العلوم المجتمع التقانات الطيران الأديان فهرس البوابات ولاية وهران (بالفرنسية: Wilaya d'Oran) (بالإنجليزية: Oran Province) (تنطق باللهجة المحلي...

2000 song by Demon & 2021 song by DJ Snake You Are My HighSingle by Demon vs. Heartbreakerfrom the EP You Are My High Released16 January 2000 (2000-01-16)Recorded1999Genre French house Length3:475:28 (Extended Version)Label 20000st Records Small Songwriter(s) Demon Heartbreaker Charlie Wilson Johnsye Andrea Smith Ronnie Wilson Producer(s)Demon, HeartbreakerDemon singles chronology Midnight Funk (1999) You Are My High (2000) Don't Make Me Cry (2002) You Are My High is a ...

Australian multicultural radio network For other uses, see SBS Radio (disambiguation). SBS RadioMelbourne and SydneyBroadcast areaAustralia (national) via AM, FM, DAB+, digital TV, online and satelliteFrequencyVariousBrandingSBS AudioProgrammingLanguage(s)EnglishVariousFormatMultilingual programmingSubchannelsSBS ChillSBS PopAsiaSBS Arabic 24SBS South AsianOwnershipOwnerSpecial Broadcasting ServiceHistoryFirst air date9 June 1975; 49 years ago (1975-06-09) (as 2EA, 3EA)[...

2024年スケートアメリカ アレン・イベントセンター大会概要英語 2024 Skate America大会種 ISUグランプリシリーズ優勝ポイント 400シーズン 2024-2025日程 10月18日 - 10月20日主催 アメリカフィギュアスケート連盟、国際スケート連盟開催国 アメリカ合衆国開催地 アレン会場 アレン・イベントセンター賞金総額 US$ 180,000.00公式サイト 公式サイト前回優勝者男子前回優勝 イ�...
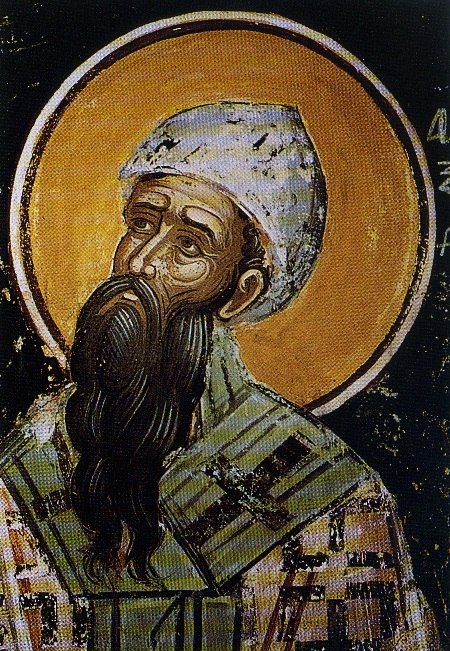
Patriarch of Alexandria from 412 to 444 For other people with the same name, see Pope Cyril of Alexandria (disambiguation). SaintCyril of AlexandriaSt Cyril of Alexandria, Patriarch, and ConfessorArchdioceseAlexandriaSeeAlexandriaPredecessorTheophilus of AlexandriaSuccessorPope Dioscorus I of AlexandriaPersonal detailsBornc. 376Didouseya, Province of Egypt, Byzantine EmpireDied444 (aged 67–68)Alexandria, Province of Egypt, Byzantine EmpireSainthoodFeast day18 January and 9 Ju...

American activist (1928–2024) For other people named James Lawson, see James Lawson (disambiguation). James LawsonLawson in 2005BornJames Morris Lawson Jr.(1928-09-22)September 22, 1928Uniontown, Pennsylvania, U.S.DiedJune 9, 2024(2024-06-09) (aged 95)Los Angeles, California, U.S.Education Baldwin Wallace University (BA) Oberlin College Vanderbilt University Boston University (STB) Occupation(s)Activist, professor, ministerKnown forNashville sit-ins James Morris Lawson Jr. (Septem...

2004 studio album by Hugh CornwellBeyond Elysian FieldsStudio album by Hugh CornwellReleased4 October 2004Recorded2003StudioLooking Glass, New YorkTruck Farm, New OrleansGenre Alternative rock post-punk Length43:29LabelInvisible Hands MusicProducer Tony Visconti Danny Kadar Hugh Cornwell chronology In the Dock(2003) Beyond Elysian Fields(2004) Hooverdam(2008) Beyond Elysian Fields is the sixth studio album by Hugh Cornwell, released by Invisible Hands Music on 4 October 2004[1 ...

1546–1547 conflict in the Holy Roman Empire For the 1552 Princes' Revolt, see Second Schmalkaldic War. Schmalkaldic WarPart of European wars of religionTitian's Equestrian Portrait of Charles V (1548) celebrates Charles's victory at the Battle of Mühlberg.Date10 July 1546 – 23 May 1547LocationHoly Roman EmpireResult Imperial victory[1] Capitulation of Wittenberg: Schmalkaldic League dissolved, Saxon electoral dignity passed to the Albertine House of WettinBelligerents Holy ...

Scottish surgeon (1795–1860) James BraidJames Braid, 1854Born(1795-06-19)19 June 1795Portmoak, Kinross-shire, ScotlandDied25 March 1860(1860-03-25) (aged 64)Chorlton-on-Medlock, Manchester, EnglandAlma materUniversity of EdinburghKnown forSurgeryhypnotismScientific careerFieldsMedicinenatural historyInstitutionsRoyal College of Surgeons of EdinburghWernerian Natural History Society Hypnosis Applications Age regression in therapy Animal magnetism Hypnotherapy Stage hypnosis Se...

Bust Eugène Auguste Nicolas Millon (24 April 1812 – 22 October 1867) was a French chemist and physician. He is remembered in the name of Millon's reagent which reacts with tyrosine in proteins to form a brown precipitate. The reagent is used for determination of the presence of soluble proteins. Millon was born in Saint-Seine-l'Abbaye and after his education, he taught briefly at the Collège Rollin after before training in medicine at the military hospital at Val-de-Grâce from 1832 to 18...