Anamorphism
|
Read other articles:

Gereja Hati Kudus Yesus, TasikmalayaGereja Katolik Hati Kudus Yesus TasikmalayaLokasiJl. Sutisna Senjaya No. 50, Kota Tasikmalaya 46113 Jawa BaratJumlah anggota/umatSekitar 1700 (menurut data SIMU 2019)Situs webhttps://www.hkytasik.org/SejarahDidirikan1947DedikasiHati Yesus yang Maha KudusAdministrasiKeuskupanBandungJumlah Imam3Imam yang bertugasRD. Fabianus Muktiyarso, PrParokialStasi3Jumlah lingkungan15 Gereja Hati Kudus Yesus Tasikmalaya adalah sebuah gereja Katolik yang masuk dalam wilaya...

هذه المقالة يتيمة إذ تصل إليها مقالات أخرى قليلة جدًا. فضلًا، ساعد بإضافة وصلة إليها في مقالات متعلقة بها. (أبريل 2015) ثوران بارذربونجا 2014-2015معلومات عامةمؤشر التفجر البركاني Volcanic explosivity index 0: Hawaiian (en) تعديل - تعديل مصدري - تعديل ويكي بيانات ثورة بركان بارذربونجا في عام 2014 هي ثور...

American politician and mayor of Jersey City since 2013 Steve Fulop49th Mayor of Jersey CityIncumbentAssumed office July 1, 2013Preceded byJerramiah HealyMember of Jersey City Councilfrom Ward EIn officeJuly 1, 2005 – June 30, 2013Preceded byJunior MaldonadoSucceeded byCandice Osborne Personal detailsBornSteven Michael Fulop (1977-02-28) February 28, 1977 (age 47)Edison, New Jersey, U.S.Political partyDemocraticSpouse Jaclyn Thompson (m. 2016)...

2005 single by Bob Sinclar featuring Gary Pine Not to be confused with Generation Love. Love GenerationSingle by Bob Sinclar featuring Gary Pinefrom the album Western Dream Released19 September 2005 (2005-09-19)Length 8:55 (album version) 3:30 (video & radio versions) 2:50 (radio edit) Label Yellow Productions Defected Songwriter(s) Duane Harden Christophe le Friant Gary Pine Jay Woodhouse JG Schreiner Alain Wisniak Producer(s)Bob SinclarBob Sinclar singles chronology Y...

Sungai Mekong (ទន្លេរមេគង្គ) Megaung Myit, แม่น้ำโขง (Maenam Khong), Mékôngk, Tonle Thom, Cửu Long, Mê Kông, 湄公 (Méigōng) Sungai Sungai Mekong. Countries Tiongkok, Burma (Myanmar), Laos, Thailand, Kamboja, Vietnam Provinsi Qinghai, Tibet, Yunnan, Shan, Luang Namtha, Bokeo, Oudomxay, Luang Prabang, Sayabouly, Vientiane, Vientiane, Bolikhamsai, Khammouane, Savannakhet, Salavan, Champasak Sumber Mata air Lasagongma - location ...

Species of virus Influenza D virus Virus classification (unranked): Virus Realm: Riboviria Kingdom: Orthornavirae Phylum: Negarnaviricota Class: Insthoviricetes Order: Articulavirales Family: Orthomyxoviridae Genus: Deltainfluenzavirus Species: Influenza D virus Influenza D virus is a species in the virus genus Deltainfluenzavirus, in the family Orthomyxoviridae, that causes influenza. Influenza D viruses are known to infect pigs and cattle; no human infections from this virus have been obser...

Dominic A. AntonelliLahir23 Agustus 1967 (umur 56)[1]Detroit, MichiganStatusPurna tugasKebangsaanAmerika SerikatAlmamaterInstitut Teknologi Massachusetts (Sarjana)Universitas Washington (Magistrat)PekerjaanPilot uji cobaKarier luar angkasaAntariksawan NASAPangkatKomandan Angkatan Laut Amerika SerikatWaktu di luar angkasa24 hari 13 jam 58 menitSeleksi2000 NASA GroupMisiSTS-119, STS-132Lambang misi Dominic Anthony Tony Antonelli (lahir 23 Agustus 1967) adalah seorang purnawirawan ...

Questa voce sull'argomento stagioni delle società calcistiche italiane è solo un abbozzo. Contribuisci a migliorarla secondo le convenzioni di Wikipedia. Segui i suggerimenti del progetto di riferimento. Voce principale: Associazione Sportiva Dilettantistica Acqui 1911. Acqui Unione SportivaStagione 1938-1939Sport calcio Squadra Acqui Allenatore Andrea Viviano Presidente Giuseppe Collino Serie C4º posto nel girone D. Coppa ItaliaPrimo turno eliminatorio. StadioCampo Sportivo Lit...

UGM-96 Trident I, atau Trident C4 adalah sebuah peluru kendali / rudal balistik Amerika yang diluncurkan Submarine, dibangun oleh Lockheed Martin Space Systems di Sunnyvale, California. Pertama digunakan pada tahun 1979, Trident I menggantikan rudal Poseidon. Ini sudah pensiun pada tahun 2005, [2] yang telah digantikan oleh Trident II. Trident I adalah tiga tahap, rudal berbahan bakar padat. Referensi Wikimedia Commons memiliki media mengenai Trident missile. lbsSistem penggolongan peluru ke...

Dutch politician and farmer (born 1979) In this Dutch name, the birth surname is Monaster and the marital name is Vedder. Eline VedderVedder (center) at a 2021 farmers' protestMember of the House of RepresentativesIncumbentAssumed office 10 May 2023Preceded byJaco GeurtsMember of the Provincial Council of DrentheIn office28 March 2019 – 30 May 2023Succeeded bySonja Hilgenga-van DamMember of the Municipal Council of De WoldenIn office29 March 2018 – 25 April 2...

Crusade fought against heretics in Bosnia Bosnian CrusadePart of the CrusadesDate1235–1241LocationBosnia, possibly also Slavonia and ZachlumiaResult Bosnian VictoryTerritorialchanges Hungarian occupation of peripheral parts of Bosnia reversed after the warBelligerents Kingdom of Hungary Banate of BosniaCommanders and leaders Coloman of Hungary Matej NinoslavvteCrusadesIdeology and institutions Crusading movement In the Holy Land (1095–1291) First 1101 Norwegian Venetian 1129 Second Th...

Auguste BravaisAuguste Bravais (c. 1850)Lahir23 Agustus 1811AnnonayMeninggal30 Maret 1863(1863-03-30) (umur 51)Le ChesnayKebangsaanPrancisAlmamaterÉcole PolytechniqueDikenal atasKisi BravaisKarier ilmiahBidangKristalografi Auguste Bravais (pengucapan bahasa Prancis: [oɡyst bʁavɛ]; 23 Agustus 1811, Annonay, Ardèche – 30 Maret 1863, Le Chesnay, Prancis) adalah seorang fisikawan Prancis yang dikenal berkat karyanya dalam kristalografi, konsep kisi Bravais, dan formulasi hukum B...
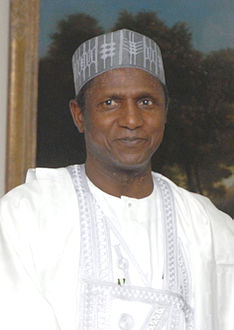
تحوي هذه المقالة أو هذا القسم ترجمة آلية. فضلًا، ساهم في تدقيقها وتحسينها أو إزالتها لأنها تخالف سياسات ويكيبيديا. (نقاش) (أبريل 2020) عمر يارادوا (بالهوسية: Umaru Musa Yar'Adua)[1] معلومات شخصية الميلاد 16 أغسطس 1951 [2][3] الوفاة 5 مايو 2010 (58 سنة) [2][3] سبب ال...
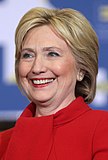
Main article: 2016 United States presidential election 2016 United States presidential election in South Dakota ← 2012 November 8, 2016 2020 → Turnout59.90% [1] Nominee Donald Trump Hillary Clinton Gary Johnson Party Republican Democratic Libertarian Home state New York New York New Mexico Running mate Mike Pence Tim Kaine Bill Weld Electoral vote 3 0 0 Popular vote 227,721 117,458 20,845 Percentage 61.53% 31.74% 5.63% County results P...
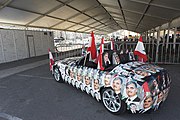
This article has multiple issues. Please help improve it or discuss these issues on the talk page. (Learn how and when to remove these template messages) This article needs additional citations for verification. Please help improve this article by adding citations to reliable sources. Unsourced material may be challenged and removed.Find sources: Automobile folklore – news · newspapers · books · scholar · JSTOR (December 2010) (Learn how and when to re...

سلمان بن سعود بن عبد العزيز آل سعود معلومات شخصية الميلاد 1953 (العمر 71 سنة)الرياض مواطنة السعودية الأب سعود بن عبد العزيز آل سعود عائلة آل سعود الحياة العملية المدرسة الأم جامعة الملك سعود المهنة موظف مدني اللغة الأم العربية اللغات العربية تعديل مصدر�...
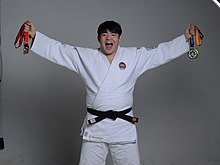
乌勒齐巴亚尔·杜伦巴亚尔出生1994年7月31日 (29歲)職業柔道家 乌勒齐巴亚尔·杜伦巴亚尔(蒙古語:Өлзийбаярын Дүүрэнбаяр,1996年1月31日—),蒙古男子柔道运动员,2014年亚洲运动会男子100公斤以上级银牌得主、2018年亚洲运动会男子100公斤以上级银牌得主、2018年世界柔道锦标赛男子100公斤以上级铜牌得主。[1] 参考资料 ^ Duurenbayar ULZIIBAYAR / IJF.org....

Kementerian Urusan Luar Negeri USSRМинистерство иностранных дел СССРSeluruh segel kementerian Uni Soviet memakai lambang SovietInformasi lembagaDibentuk16 July 1923Dibubarkan15 November 1991Lembaga penggantiKementerian Urusan Luar Negeri Federasi Rusia (1992)Wilayah hukumUni Republik Sosialis SovietKantor pusatMoskwa, SFSR Rusia, Uni Soviet Kementerian Urusan Luar Negeri Uni Republik Sosialis Soviet (bahasa Rusia: Министерство иностранны...

Mappa del Territorio Antartico Britannico; in blu, la terra della Regina Elisabetta La Terra della Regina Elisabetta è la parte del Territorio Antartico Britannico che si estende dal Mare di Weddell al Polo Sud. Il territorio era senza nome fino al 18 dicembre 2012. Indice 1 Storia 2 Descrizione 3 Note 4 Voci correlate 5 Altri progetti Storia In occasione di una visita della Regina Elisabetta al Foreign Office a Londra, il 18 dicembre 2012, fu annunciato che una superficie di 169.000 miglia ...

British Army Officer (1777–1853) George BurrellBorn26 February 1777Longhoughton, Northumberland, EnglandDied4 January 1853 (aged 75)Alnwick, Northumberland, EnglandAllegianceUnited KingdomService/branchBritish ArmyRankLieutenant-GeneralWarsNapoleonic WarsWar of 1812First Anglo-Chinese WarAwardsCompanion of the Order of the Bath Lieutenant-General George Burrell CB (26 February 1777 – 4 January 1853) was a British Army officer. He served in the Napoleonic Wars (1803–1815), War of 1812, a...